javamail编写邮件收发项目的具体代码,并解决javax.mail.AuthenticationFailedException: 530 Login fail. A secure connection is requiered(such as ssl)这个问题的具体做法
时间: 2024-10-25 15:06:35 浏览: 45
在JavaMail中,发送邮件时可能会遇到`AuthenticationFailedException`,特别是当SMTP服务器需要安全连接(如SSL/TLS)而未正确配置时。解决这个问题的具体做法如下:
首先,你需要确认SMTP服务器是否支持SSL/TLS并且需要客户端证书(如果有)。如果没有,你可以尝试使用非SSL连接,但这种方式可能存在安全风险。
下面是使用SSL连接示例的代码片段:
```java
import javax.mail.*;
import javax.mail.internet.*;
import java.util.Properties;
public class EmailSender {
public static void main(String[] args) {
String host = "smtp.example.com"; // 替换为你的SMTP服务器地址
int port = 465; // 通常是465 for SSL or 587 for STARTTLS
String username = "your_email@example.com";
String password = "your_password";
Properties props = System.getProperties();
props.put("mail.smtp.starttls.enable", "true"); // 如果SMTP需要STARTTLS,则设置此属性为true
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.socketFactory.port", port);
props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory");
Session session = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(username));
InternetAddress[] recipients = {new InternetAddress("recipient@example.com")};
message.setRecipients(Message.RecipientType.TO, recipients);
message.setSubject("Test email");
message.setText("This is a test email using JavaMail with SSL.");
Transport.send(message);
System.out.println("Email sent successfully.");
} catch (MessagingException e) {
e.printStackTrace(); // 打印异常以便于调试
if (e instanceof AuthenticationFailedException && ((AuthenticationFailedException) e).getMessage().contains("530")) {
// 如果是由于SSL/TLS认证失败,可以尝试关闭SSL/TLS并再次尝试
props.remove("mail.smtp.starttls.enable");
props.put("mail.smtp.socketFactory.port", 25); // 将端口更改为无安全连接的SMTP端口
session = Session.getInstance(props, new Authenticator());
// 然后重新发送
// ...
}
}
}
}
```
在这个例子中,我们首先尝试启用STARTTLS,如果失败就切换到非SSL连接(注意,这并不是最佳实践,仅用于示例目的)。实际应用中,你应该确保SMTP服务器确实在SSL/TLS模式下工作,并提供正确的证书和凭据。
阅读全文
相关推荐
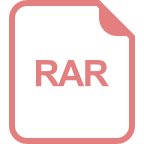
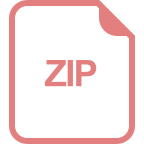
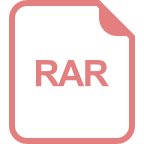

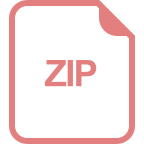
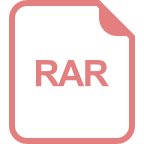
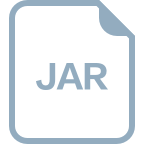
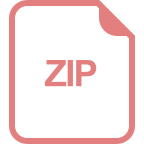
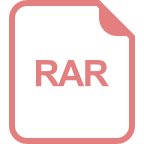
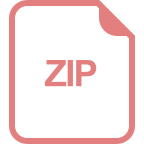
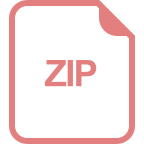
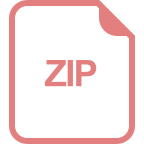
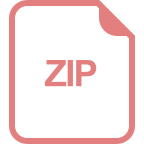
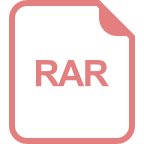
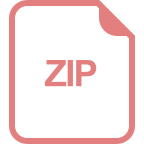
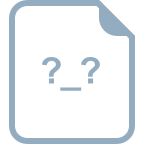
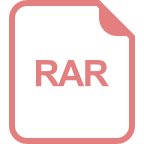
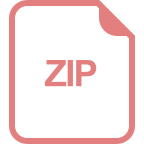