编写一个函数,该函数接收一个整数数组和数组的大小作为参数,并返回指向数组中最大元素的指针
时间: 2024-11-09 07:27:59 浏览: 9
编写一个函数来找到整数数组中的最大元素并返回其指针,可以使用如下伪代码表示:
```cpp
// 假设我们叫这个函数findMaxElement
void* findMaxElement(int* arr, int size) {
// 初始化一个假设的最大值和它的指针
int* max = arr;
int maxValue = arr[0];
// 遍历数组,如果当前元素大于maxValue,更新max和maxValue
for (int i = 1; i < size; ++i) {
if (arr[i] > maxValue) {
max = &arr[i]; // 更新最大值的指针
maxValue = arr[i]; // 保持最大值不变
}
}
return max; // 返回指向最大元素的指针
}
```
在这个函数中,我们首先将`max`指针设置为第一个元素,然后逐个检查后续元素,如果发现更大的元素,就更新`max`和`maxValue`。最后返回指向最大元素的指针。
相关问题
编写一个C语言函数,该函数接受一个整数数组和数组的大小,并通过指针返回数组中的最大值
你可以编写一个名为`find_max`的C语言函数,它接收一个整数数组`arr`的地址(也就是指针)和数组的长度`size`。下面是一个简单的示例:
```c
#include <stdio.h>
#include <limits.h> // 引入INT_MAX以便获取最大的整数值
// 函数声明
int find_max(int* arr, size_t size);
int main() {
int array[] = {5, 9, 2, 7, 1, 8};
size_t array_size = sizeof(array) / sizeof(array[0]);
int max_value = find_max(array, array_size);
printf("数组中的最大值是: %d\n", max_value);
return 0;
}
// 函数实现
int find_max(int* arr, size_t size) {
int max_val = INT_MIN; // 初始化为负无穷大
for (size_t i = 0; i < size; i++) {
if (arr[i] > max_val) {
max_val = arr[i];
}
}
return max_val;
}
```
在这个例子中,`find_max`函数遍历整个数组,每次都检查当前元素是否大于之前找到的最大值。如果是,就更新最大值。最后,函数返回找到的最大值。
编写一个C语言函数,该函数接受一个整数数组和数组的大小,并通过指针返回数组中的最大值。需要有注释语句
```c
#include <stdio.h> // 引入标准输入输出库
// 定义一个名为find_max的函数,它接收一个整数数组和数组长度作为参数
int find_max(int* array, int size) {
// 使用变量max初始化为数组的第一个元素
int max = array[0];
// 通过遍历数组(从索引1开始),将当前元素与已知的最大值比较
// 如果找到更大的元素,则更新max
for (int i = 1; i < size; i++) {
if (array[i] > max) { // 当前元素大于最大值
max = array[i]; // 更新最大值
}
}
// 返回发现的最大值
return max;
}
int main() {
int numbers[] = {5, 9, 1, 7, 3}; // 示例数组
int length = sizeof(numbers) / sizeof(numbers[0]); // 计算数组长度
int max_value = find_max(numbers, length); // 调用find_max函数并存储结果
printf("数组中的最大值是: %d\n", max_value); // 输出结果
return 0;
}
```
以上代码定义了一个名为`find_max`的函数,它通过指针遍历数组,找到最大的整数值。关键部分有明确的注释说明了函数的工作流程和变量的作用。在`main`函数中,我们创建了一个示例数组并调用了`find_max`获取最大值。
阅读全文
相关推荐
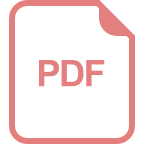
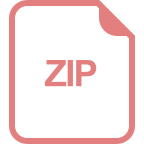
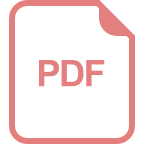
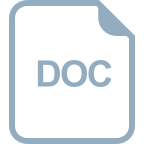
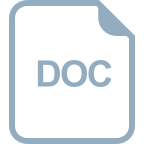











