怎么导naive-ui的style
时间: 2024-04-22 11:24:49 浏览: 13
要导入 Naive UI 的样式,你需要按照以下步骤操作:
1. 首先,确保你已经安装了 Naive UI。可以通过 npm 或者 yarn 进行安装,具体命令如下:
```
npm install naive-ui
```
或者
```
yarn add naive-ui
```
2. 在你的项目中,找到入口文件(通常是 `main.js` 或者 `index.js`),在文件的开头添加以下代码:
```javascript
import { createApp } from 'vue'
import naive from 'naive-ui'
import 'naive-ui/lib/style.css'
const app = createApp(App)
app.use(naive)
app.mount('#app')
```
这段代码会将 Naive UI 的样式导入到你的项目中。
3. 现在,你可以在你的 Vue 组件中使用 Naive UI 的组件和样式了。例如,在一个 Vue 组件中使用一个按钮:
```vue
<template>
<n-button>按钮</n-button>
</template>
```
这样,你就成功导入了 Naive UI 的样式,并可以在你的项目中使用它了。记得根据你的项目配置进行相应的修改。
相关问题
naive-ui弹出框
Naive-UI 是一款基于 Vue.js 的 UI 组件库,它提供了弹出框(Dialog)组件用于显示模态框。要使用 Naive-UI 的弹出框,你需要先安装并引入 Naive-UI 的相关依赖。
以下是一个使用 Naive-UI 弹出框的简单示例:
1. 首先,确保你已经安装了 Vue.js 和 Naive-UI:
```bash
npm install vue@2.x naive-ui
```
2. 在你的 Vue.js 项目中,引入 Naive-UI 的样式和组件:
```javascript
import { createApp } from 'vue';
import naive from 'naive-ui';
import 'naive-ui/lib/index.css';
const app = createApp();
app.use(naive);
app.mount('#app');
```
3. 在你的组件中使用弹出框组件:
```html
<template>
<div>
<n-button @click="showDialog">点击弹出框</n-button>
<n-dialog v-model:visible="dialogVisible">
<span>这是一个弹出框</span>
<template #footer>
<n-button @click="dialogVisible = false">关闭</n-button>
</template>
</n-dialog>
</div>
</template>
<script>
export default {
data() {
return {
dialogVisible: false,
};
},
methods: {
showDialog() {
this.dialogVisible = true;
},
},
};
</script>
```
上述示例中,我们使用了 Naive-UI 的 Button 组件来触发弹出框的显示,使用了 Dialog 组件来创建弹出框,通过 v-model:visible 来控制弹出框的显示与隐藏。在弹出框的底部,我们通过 #footer 插槽自定义了一个关闭按钮。
你可以根据自己的需求进一步定制和使用 Naive-UI 弹出框组件。
naive-ui的穿梭框
naive-ui是一个基于Vue 3的UI框架,它提供了一些常用的组件,包括穿梭框(Transfer)组件。穿梭框可以让用户在两个列表之间移动元素,非常适合用于数据筛选、分类等场景。
在naive-ui中,使用穿梭框非常简单,只需要引入Transfer组件并传入相应的数据即可。例如:
```html
<n-transfer
:data="data"
v-model="value"
:titles="['Source', 'Target']"
/>
```
其中,`data`是一个数组,包含了所有可供选择的元素;`value`是一个数组,表示已经被选中的元素;`titles`是一个数组,表示两个列表的标题。
相关推荐
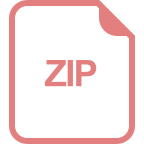












