给出世界六大城市之间的航线距离(英里),试确定从北京出发飞遍每 座城市回到北京的最短总航线。
时间: 2024-04-17 17:27:39 浏览: 63
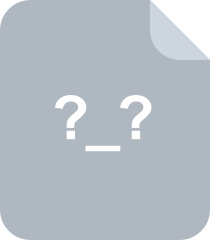
求两个城市之间的最短路径
为了确定从北京出发飞往每座城市并回到北京的最短总航线,我们可以使用旅行商问题(TSP)来解决。TSP是一个经典的组合优化问题,可以使用启发式算法来逼近最优解。在这里,我们可以使用遗传算法来求解。
下面是一个使用遗传算法解决TSP问题的示例代码:
```python
import random
# 定义城市列表
cities = ["北京", "伦敦", "纽约", "东京", "巴黎", "悉尼"]
# 定义城市之间的距离矩阵
distances = [[0, 5188, 6886, 2118, 5693, 5765],
[5188, 0, 3465, 5981, 343, 10561],
[6886, 3465, 0, 6761, 3631, 9935],
[2118, 5981, 6761, 0, 9639, 4779],
[5693, 343, 3631, 9639, 0, 10590],
[5765, 10561, 9935, 4779, 10590, 0]]
# 定义遗传算法参数
population_size = 50
elite_size = 10
mutation_rate = 0.01
generations = 100
# 创建一个初始种群
def create_initial_population():
population = []
for _ in range(population_size):
population.append(random.sample(cities, len(cities)))
return population
# 计算路径的总距离
def calculate_total_distance(path):
total_distance = 0
for i in range(len(path)-1):
start_city = path[i]
end_city = path[i+1]
total_distance += distances[cities.index(start_city)][cities.index(end_city)]
total_distance += distances[cities.index(path[-1])][cities.index(path[0])]
return total_distance
# 交叉操作(部分映射交叉)
def crossover(parent1, parent2):
child = [None] * len(parent1)
start_index = random.randint(0, len(parent1)-2)
end_index = random.randint(start_index+1, len(parent1)-1)
for i in range(start_index, end_index+1):
child[i] = parent1[i]
for i in range(len(parent2)):
if parent2[i] not in child:
for j in range(len(child)):
if child[j] is None:
child[j] = parent2[i]
break
return child
# 变异操作(交换两个位置的城市)
def mutate(path):
index1 = random.randint(0, len(path)-1)
index2 = random.randint(0, len(path)-1)
path[index1], path[index2] = path[index2], path[index1]
return path
# 选择操作(锦标赛选择)
def selection(population):
selected = []
for _ in range(elite_size):
selected.append(min(population, key=calculate_total_distance))
population.remove(selected[-1])
for _ in range(population_size - elite_size):
tournament = random.sample(population, 3)
selected.append(min(tournament, key=calculate_total_distance))
return selected
# 进化过程
def evolve(population):
selected = selection(population)
offspring = []
for _ in range(elite_size):
offspring.append(selected[_])
for _ in range(population_size - elite_size):
parent1 = random.choice(selected)
parent2 = random.choice(selected)
child = crossover(parent1, parent2)
if random.random() < mutation_rate:
child = mutate(child)
offspring.append(child)
return offspring
# 主函数
def main():
population = create_initial_population()
for _ in range(generations):
population = evolve(population)
best_path = min(population, key=calculate_total_distance)
shortest_distance = calculate_total_distance(best_path)
best_path.append(best_path[0]) # 添加回到起点的路径
print("最短总航线: ", best_path)
print("最短总距离: ", shortest_distance)
if __name__ == '__main__':
main()
```
请注意,上述代码中的距离矩阵是根据您提供的城市之间的距离进行填充的。您可以根据实际情况进行修改和扩展。
阅读全文
相关推荐
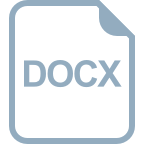
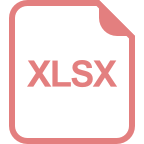
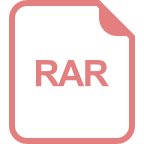
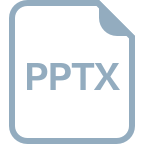
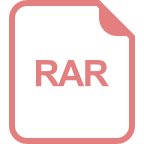
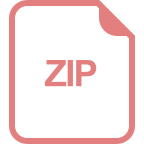
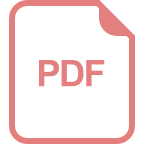
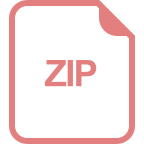
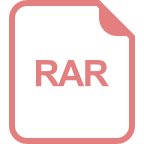
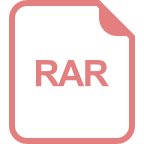
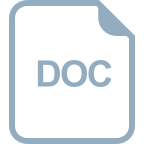
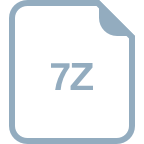
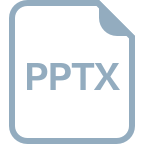
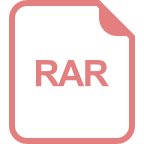