tensorflow简单网络搭建
时间: 2024-09-09 09:03:58 浏览: 45
在TensorFlow中,构建一个简单的神经网络通常涉及以下几个步骤:
1. **导入库和模块**:
```python
import tensorflow as tf
from tensorflow.keras.layers import Dense, Input
from tensorflow.keras.models import Model
```
2. **定义输入层和模型结构**:
使用`Input`函数定义输入张量,并指定其形状。例如,如果处理的是二维数组(如图像),我们可以这样定义:
```python
input_shape = (height, width, channels) # 根据实际需求替换
inputs = Input(shape=input_shape)
```
然后添加隐藏层和输出层。对于一个有两层隐层和一个单个节点的输出层的全连接网络,可以这样编写:
```python
hidden_layer_1 = Dense(units=units1, activation='relu')(inputs)
hidden_layer_2 = Dense(units=units2, activation='relu')(hidden_layer_1)
outputs = Dense(units=1, activation='sigmoid') # 如果任务是二分类,激活函数可能是'softmax'
```
3. **创建完整模型**:
将隐藏层和输出层连接起来构成完整的模型。Keras会自动处理权重的初始化和反向传播:
```python
model = Model(inputs=inputs, outputs=outputs)
```
4. **编译模型**:
设置优化器、损失函数和评估指标:
```python
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # 对于二分类问题
```
5. **训练模型**:
使用`fit`函数提供训练数据、标签以及可能的其他超参数:
```python
history = model.fit(x_train, y_train, epochs=num_epochs, batch_size=batch_size, validation_split=0.2)
```
6. **评估模型**:
使用`evaluate`函数对测试集进行性能评估:
```python
test_loss, test_accuracy = model.evaluate(x_test, y_test)
```
注意这里的参数需要根据具体的任务(如图像分类、回归等)、数据集大小和复杂度进行调整。
阅读全文
相关推荐
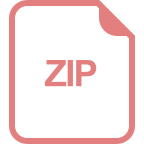
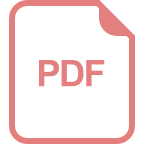
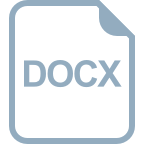
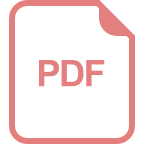
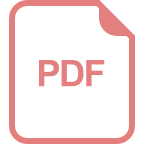
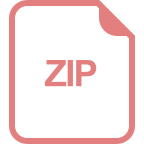
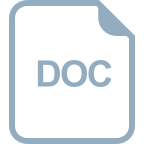
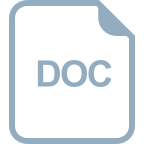
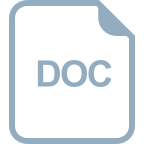
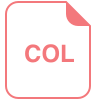






