使用pytorch写一个deeplabV3
时间: 2024-03-30 08:07:16 浏览: 150
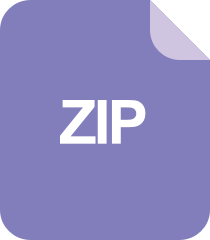
deeplabv3图像语义分割源码,pytorch框架实现,一键运行
当然可以!以下是使用 PyTorch 编写 DeepLabV3 模型的基本代码示例:
```python
import torch
import torch.nn as nn
import torchvision.models as models
# 定义 DeepLabV3 模型
class DeepLabV3(nn.Module):
def __init__(self, num_classes):
super(DeepLabV3, self).__init__()
self.resnet = models.resnet50(pretrained=True)
self.aspp = ASPP()
self.decoder = Decoder(num_classes)
def forward(self, x):
x = self.resnet.conv1(x)
x = self.resnet.bn1(x)
x = self.resnet.relu(x)
x = self.resnet.maxpool(x)
x = self.resnet.layer1(x)
x = self.resnet.layer2(x)
x = self.resnet.layer3(x)
x = self.resnet.layer4(x)
x = self.aspp(x)
x = self.decoder(x)
return x
# 定义 ASPP 模块
class ASPP(nn.Module):
def __init__(self):
super(ASPP, self).__init__()
# 定义具体的 ASPP 模块结构
def forward(self, x):
# ASPP 前向传播逻辑
return x
# 定义解码器模块
class Decoder(nn.Module):
def __init__(self, num_classes):
super(Decoder, self).__init__()
# 定义具体的解码器模块结构
def forward(self, x):
# 解码器前向传播逻辑
return x
# 创建 DeepLabV3 模型实例
num_classes = 21 # 类别数量
model = DeepLabV3(num_classes)
# 使用模型进行训练或推理
input_tensor = torch.randn(1, 3, 224, 224) # 输入张量
output_tensor = model(input_tensor)
```
请注意,上述代码只是一个基本的框架,仅包含模型的结构定义。您需要根据 DeepLabV3 论文和具体需求,实现 ASPP 模块和解码器模块的具体结构和前向传播逻辑。此外,您还需要根据您的数据集修改最后一层解码器的输出通道数,以适应您的分类任务。
希望这可以帮助您入门 DeepLabV3 的 PyTorch 实现!如果您有更多问题,请随时提问。
阅读全文
相关推荐
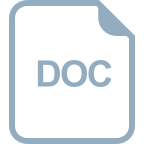
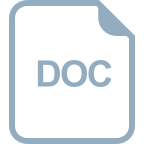

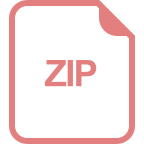
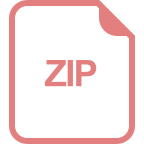
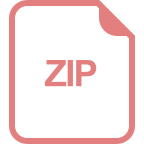
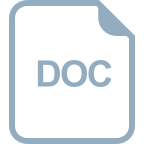









