C++如何判断现有值是不是set里倒数第二个数
时间: 2024-10-20 08:12:56 浏览: 13
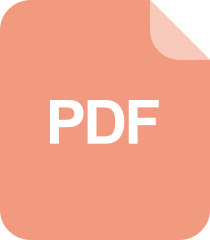
C++程序设计课件 ch9 类与对象(二)

在C++中,如果你有一个`std::set`容器,想要检查当前元素是否是它的倒数第二个元素,你可以通过以下步骤实现:
1. 首先,你需要获取集合的大小(即元素的数量)。这可以使用`size()`函数完成。
2. 然后,你可以检查这个大小是否大于1,因为如果集合只有一个元素,就没有倒数第二个元素的概念。
3. 接下来,你可以遍历集合,从最后一个元素开始向前查找。你可以使用迭代器(如`std::set::rbegin()`返回逆序迭代器),然后逐个移动,直到找到倒数第二个元素。
下面是一个简单的示例代码片段,假设`mySet`是你需要检查的`std::set`:
```cpp
#include <iostream>
#include <set>
bool isSecondToLastElement(const std::set<int>& mySet) {
if (mySet.size() <= 1) {
return false; // 如果集合小于等于1个元素,没有倒数第二个
}
auto it = mySet.rbegin(); // 从后往前迭代
++it; // 跳过第一个元素
return *it == your_value; // 比较当前元素是否为你提供的值
}
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
int targetValue = 3;
bool isSecondToLast = isSecondToLastElement(mySet);
if (isSecondToLast) {
std::cout << "Your value is the second to last in the set." << std::endl;
} else {
std::cout << "Your value is not the second to last in the set." << std::endl;
}
return 0;
}
```
在这个例子中,`your_value`应该是你想要检查的那个值。
阅读全文
相关推荐
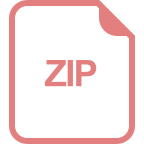
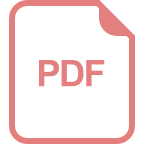

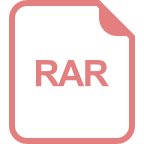
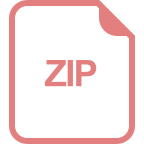
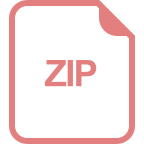
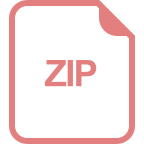
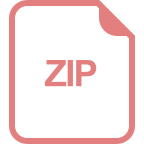
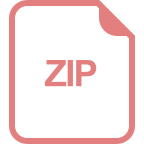
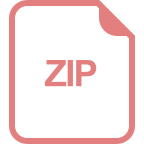
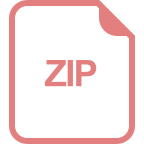
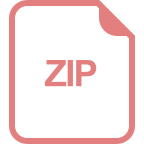