用C++写一个算法,实现多项式乘法,并分析该算法的时间复杂性。
时间: 2024-10-10 21:06:29 浏览: 24
在C++中,可以使用动态数组或矩阵来表示多项式,并使用Karatsuba算法或Horner's method(霍纳法则)来进行多项式的乘法。这里我将展示如何使用霍纳法则实现,因为它的理解起来相对直观,时间复杂性也为O(n^2),其中n是多项式的最高次数。
```cpp
#include <vector>
using namespace std;
// 定义多项式项
struct PolynomialTerm {
int coefficient;
int exponent;
};
// 多项式乘法的霍纳法则实现
PolynomialTerm polynomialMultiplication(PolynomialTerm a[], PolynomialTerm b[], int aDegree, int bDegree) {
// 初始化结果多项式的系数和指数
PolynomialTerm result = {0, 0};
// 对于每个b多项式的系数
for (int i = 0; i <= bDegree; ++i) {
// 对于a多项式的每个系数,对应乘积并累加到结果
for (int j = 0; j <= aDegree; ++j) {
result.coefficient += a[j].coefficient * pow(b[i].coefficient, (j + i));
// 更新指数
result.exponent += j + i;
}
}
return result;
}
// 示例
int main() {
PolynomialTerm polyA[] = {{1, 2}, {3, 4}, {5, 6}}; // 多项式 A = x^2 + 3x + 5
PolynomialTerm polyB[] = {{7, 8}, {9, 10}}; // 多项式 B = 7x + 9
int aDegree = sizeof(polyA) / sizeof(polyA[0]) - 1;
int bDegree = sizeof(polyB) / sizeof(polyB[0]) - 1;
PolynomialTerm result = polynomialMultiplication(polyA, polyB, aDegree, bDegree);
cout << "Product: (" << result.coefficient << ")x^" << result.exponent << endl;
return 0;
}
```
在这个例子中,输入是两个多项式的系数和它们的次数。霍纳法则实际上是一种递归的方式,每次只计算当前位的乘积,而不是全部展开后再相乘。然而,由于每个系数都需要与其他所有系数相乘一次,所以总时间复杂度为O(n^2)。
相关推荐
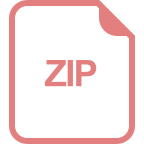
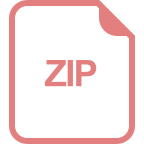
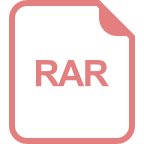














