用标准库,设计一个基于stm32的编码器测速程序,并写出主函数
时间: 2024-09-29 10:02:29 浏览: 31
在STM32微控制器上编写一个基于编码器测量转速的程序通常涉及到硬件连接、中断管理和计数器的使用。这里是一个基本的示例,假设我们使用了STM32F103系列的定时器和外部编码器作为输入。请注意,这需要对STM32的HAL库有基本了解,以及C语言编程经验。
首先,你需要包含必要的头文件,并初始化相关的硬件资源:
```c
#include "stm32f1xx_hal.h"
#include "usart.h"
// 初始化定时器和串口通信
void init_encoder_timer(void) {
TIM_HandleTypeDef htim;
USART_HandleTypeDef husart;
// 初始化定时器
htim.Instance = TIM2; // 根据实际使用选择合适的定时器
htim.Init.Prescaler = ...; // 设置预分频值
htim.Init.CounterMode = TIM_COUNTERMODE_UP;
htim.Init.Period = ...; // 设置周期长度
HAL_TIM_Init(&htim);
HAL_TIM_MspInit(&htim);
// 初始化串口通信
husart.Instance = USART2;
// ...
HAL_UART_Init(&husart);
}
void encoder_isr(TIM_HandleTypeDef *htim) {
static uint16_t last_count = 0;
// ... 在中断服务函数里读取编码器位置
uint16_t current_count = ...;
if (current_count != last_count) {
float speed = (float)(current_count - last_count) / htim->Instance->CNT / (your_encoder_resolution);
// 打印速度到串口
HAL_UART_Transmit(&husart, (uint8_t*) &speed, sizeof(speed), HAL_MAX_DELAY);
last_count = current_count;
}
}
```
主函数(`main.c`)会设置中断服务函数,开启定时器,并监听中断:
```c
int main(void) {
HAL_Init();
SystemClock_Config();
// 初始化定时器和串口
init_encoder_timer();
// 配置定时器中断
__TIM2_CLK_ENABLE();
HAL_TIM_IRQHandler(&htim);
HAL_NVIC_EnableIRQ(TIM2_IRQn); // 启动定时器2的中断
while (1) {
// 等待中断触发或执行其他任务...
}
}
// 中断向量表配置
void TIM2_IRQHandler(void) {
encoder_isr(&htim);
}
```
阅读全文
相关推荐
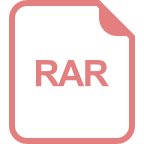
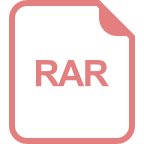
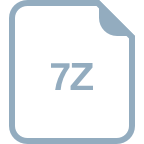
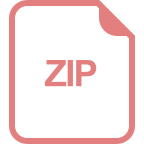
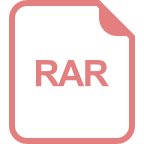
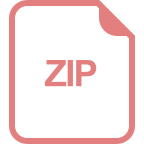
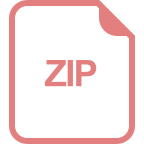
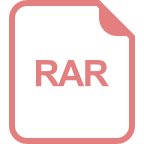
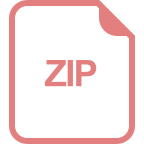
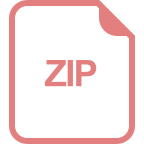
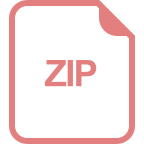
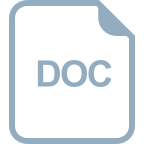