利用随机数产生20个数的列表,其中数据范围-100~100,然后用双边快速排序法对这个列表进行排序,Python
时间: 2024-12-16 13:30:56 浏览: 4
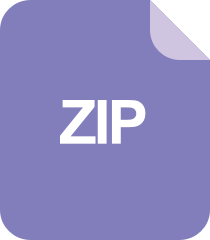
随机数排序_20个随机数_数组排序_源码

在Python中,你可以使用`random`模块生成指定范围内的随机数,并结合双边快速排序算法(也称为双轴快速排序或二分快速排序)来进行排序。这里是一个简单的步骤示例:
首先,导入需要的库:
```python
import random
```
接着,创建一个函数用于生成随机数并填充列表:
```python
def generate_random_list(length):
return [random.randint(-100, 100) for _ in range(length)]
```
然后,实现双边快速排序算法:
```python
def quicksort(arr, low=None, high=None):
if low is None and high is None:
low = 0
high = len(arr) - 1
if low < high:
pivot_index = partition(arr, low, high)
quicksort(arr, low, pivot_index - 1)
quicksort(arr, pivot_index + 1, high)
def partition(arr, low, high):
pivot = arr[low]
i = low + 1
j = high
while True:
while i <= j and arr[i] <= pivot:
i += 1
while i <= j and arr[j] >= pivot:
j -= 1
if i <= j:
arr[i], arr[j] = arr[j], arr[i]
else:
break
arr[low], arr[j] = arr[j], arr[low]
return j
```
现在,我们可以生成列表并对其进行排序:
```python
random_list = generate_random_list(20)
quicksort(random_list)
print("Sorted list:", random_list)
```
阅读全文
相关推荐
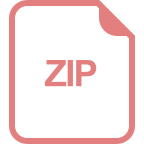
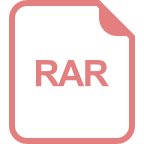

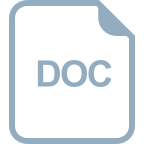
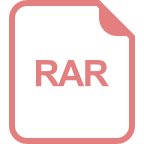
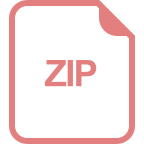
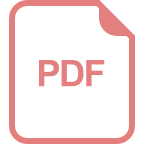
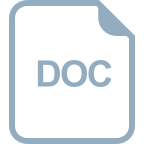
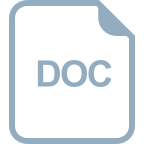
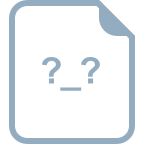
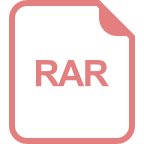
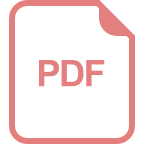
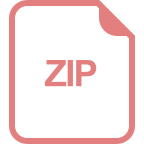
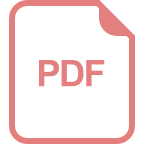
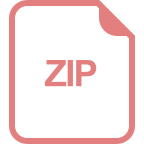