谈谈c++中的文件读取错误处理操作
时间: 2023-10-16 07:10:47 浏览: 286
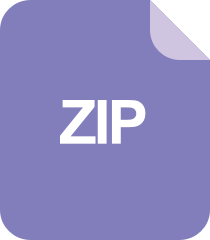
C++ 错误处理
在C++中,文件读取时可能会出现各种错误,例如文件不存在、文件权限不足、文件格式错误等。为了处理这些错误,我们可以使用异常处理机制或者检查文件流的状态。下面是两种常见的文件读取错误处理方法:
1. 异常处理:
```cpp
#include <iostream>
#include <fstream>
#include <stdexcept>
int main() {
std::ifstream infile("nonexistent_file.txt"); // 打开不存在的文件
try {
if (!infile) {
throw std::runtime_error("Failed to open the file!"); // 抛出运行时异常
}
// 读取文件内容
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl;
}
infile.close();
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl; // 输出异常信息
}
return 0;
}
```
在上述代码中,我们尝试打开一个不存在的文件"nonexistent_file.txt"。如果文件打开失败,会抛出一个`std::runtime_error`类型的异常,异常信息为"Failed to open the file!"。在`catch`块中,我们捕获并处理异常,输出错误信息到标准错误流。
2. 检查文件流状态:
```cpp
#include <iostream>
#include <fstream>
int main() {
std::ifstream infile("nonexistent_file.txt"); // 打开不存在的文件
if (!infile) {
std::cerr << "Failed to open the file!" << std::endl; // 输出错误信息
return 1;
}
// 读取文件内容
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl;
}
infile.close();
return 0;
}
```
在上述代码中,我们同样尝试打开一个不存在的文件"nonexistent_file.txt"。如果文件打开失败,文件流对象`infile`的状态将为`false`。我们可以通过检查文件流对象的状态来判断文件是否打开成功。如果文件打开失败,我们输出错误信息到标准错误流,并返回一个非零值来表示程序出错。
无论是使用异常处理还是检查文件流状态,处理文件读取错误时都需要进行适当的错误处理。这样可以提高程序的健壮性,并及时反馈给用户发生了什么问题。
阅读全文
相关推荐


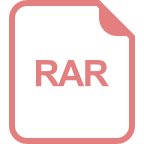
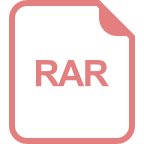
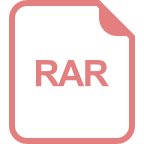
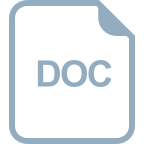
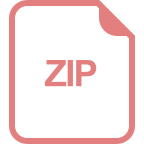
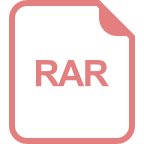
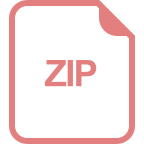
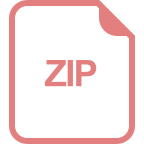
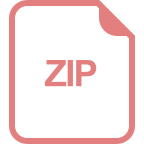
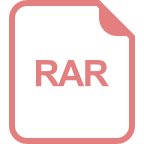
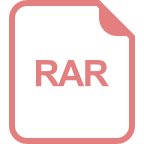
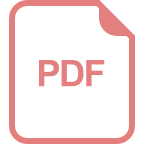
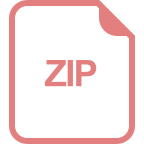
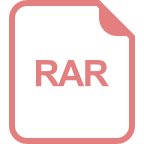