c++编程:输入:1101011011,采用CRC的生成多项式为P(X)=X4+X+1,输出冗余码1110。编程实现求冗余码的过程。
时间: 2024-09-23 21:07:19 浏览: 53
在C++中,计算CRC(循环冗余校验)的过程通常涉及位操作和模运算。首先,你需要将输入字符串转换成二进制数,然后应用CRC算法得到冗余码。以下是简化的步骤:
```cpp
#include <bitset>
#include <iostream>
// 定义CRC生成多项式
const std::string crc_poly = "10001"; // X^4 + X + 1,注意这里的字符串表示为按位存储的
// 将字符串转为二进制并计算 CRC
std::string calculate_crc(const std::string &input) {
std::bitset<32> input_bits(input, 2); // 转换为二进制形式
int polynomial = 0; // 初始化CRC值为0
for (char bit : input_bits) {
if (bit == '1') {
polynomial ^= static_cast<int>(crc_poly, 2); // 取反并异或
}
for (int i = 0; i < crc_poly.size(); ++i) { // 对每个生成多项式的位做移位和AND操作
if ((polynomial >> (crc_poly.size() - 1 - i)) & 1)
polynomial ^= static_cast<int>(crc_poly.substr(i, 1), 2);
}
}
// 返回CRC作为二进制字符串
return std::bitset<8>(polynomial).to_string();
}
int main() {
std::string input = "1101011011";
std::string result = calculate_crc(input);
std::cout << "Input: " << input << "\n";
std::cout << "CRC: " << result << "\n";
// 输出冗余码
std::cout << "Redundant code: ";
for (size_t i = result.length(); i < 8; ++i) {
result += '1';
}
std::cout << result << "\n";
return 0;
}
```
这段代码首先将输入字符串转换为二进制`input_bits`,然后通过一系列位操作计算CRC值。最后,在输出冗余码时,因为题目要求长度为8位,所以不足的部分补全为'1'。
阅读全文
相关推荐
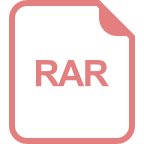
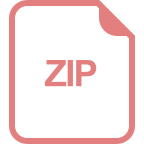
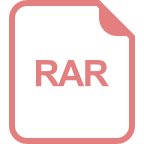















