使用fastapi 与mysql 和redis ,fastapi的框架结构如何设计
时间: 2024-05-28 18:07:27 浏览: 281
FastAPI 是一个高性能、易于学习、快速构建 Web API 的 Python 框架。FastAPI 是基于 Starlette 框架构建的,并且支持 Python 3.6+。FastAPI 的特点在于具有出色的性能和开发效率,同时提供了快速、易于使用的 API 构建工具。
使用 FastAPI 连接 MySQL 和 Redis 数据库非常简单。你可以使用异步库如 aiohttp 来连接 MySQL 和 Redis。具体来说,你可以使用 aiomysql 和 aioredis 库来连接 MySQL 和 Redis。下面是一个示例代码:
```
from fastapi import FastAPI
import aiomysql
import aioredis
app = FastAPI()
# Connect to MySQL
async def get_mysql_conn():
conn = await aiomysql.connect(
host="localhost",
port=3306,
user="root",
password="password",
db="test",
autocommit=True,
)
return conn
# Connect to Redis
async def get_redis_conn():
redis = await aioredis.create_redis_pool("redis://localhost")
return redis
@app.get("/")
async def root():
# Get MySQL connection
mysql_conn = await get_mysql_conn()
# Get Redis connection
redis_conn = await get_redis_conn()
# Perform database operations here
# Close database connections
mysql_conn.close()
redis_conn.close()
return {"message": "Hello World"}
```
在 FastAPI 中,你可以使用 Pydantic 模块定义请求和响应模型。同时,FastAPI 也支持使用依赖注入来管理数据库连接等资源。这样可以更加方便地编写可维护的代码。
FastAPI 的框架结构可以按照以下方式设计:
1. main.py:程序入口,包含 FastAPI 应用程序的初始化和配置。
2. routers 目录:包含所有路由定义和处理函数。
3. models 目录:包含所有请求和响应模型定义。
4. dependencies 目录:包含所有依赖项定义和依赖项注入逻辑。
5. services 目录:包含所有业务逻辑处理函数,如数据库操作、业务计算等。
阅读全文
相关推荐
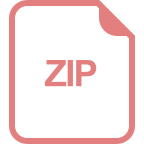
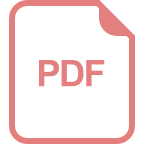
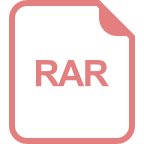
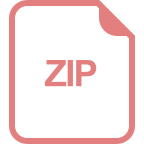
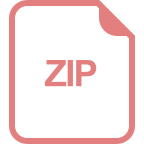
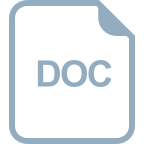
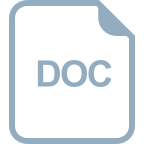
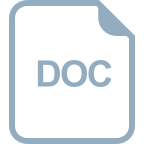
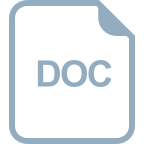
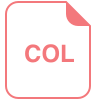
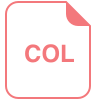
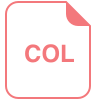
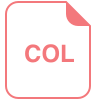
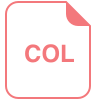
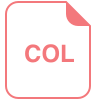
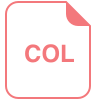
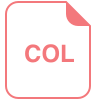
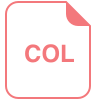
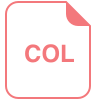
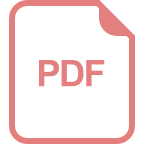