自动拨号Python
时间: 2023-11-05 10:51:12 浏览: 219
要使用Python进行自动拨号,你可以使用第三方库,如`pyautogui`或`selenium`。下面是使用这两个库的例子:
使用pyautogui库:
```python
import pyautogui
import time
# 等待2秒,给你时间将光标移到拨号程序上
time.sleep(2)
# 输入拨号号码
pyautogui.typewrite("1234567890")
# 模拟按下回车键进行拨号
pyautogui.press("enter")
```
使用selenium库(需要安装selenium和相应的浏览器驱动):
```python
from selenium import webdriver
# 创建浏览器驱动(这里以Chrome为例)
driver = webdriver.Chrome()
# 打开拨号页面
driver.get("https://example.com/dialer")
# 找到拨号输入框并输入号码
number_input = driver.find_element_by_id("dialer-input")
number_input.send_keys("1234567890")
# 找到拨号按钮并点击
dial_button = driver.find_element_by_id("dial-button")
dial_button.click()
# 关闭浏览器驱动
driver.quit()
```
请根据你的具体需求选择合适的方法,并根据实际情况修改代码。
相关问题
python实现自动拨号
要实现自动拨号,你需要使用Python的第三方库pyautogui,它可以模拟键鼠操作。以下是一个简单的自动拨号脚本示例:
```python
import pyautogui
import time
# 点击“开始菜单”
pyautogui.press('win')
time.sleep(1)
# 输入“拨号”并打开拨号器
pyautogui.typewrite('拨号')
time.sleep(1)
pyautogui.press('enter')
time.sleep(1)
# 输入电话号码并拨号
pyautogui.typewrite('10086')
time.sleep(1)
pyautogui.press('enter')
```
在这个示例中,我们使用了pyautogui来模拟了打开开始菜单、输入“拨号”并打开拨号器、输入电话号码并拨号的操作。你可以根据自己的需求进行修改和扩展。值得注意的是,这个脚本只是一个示例,实际应用中需要注意安全性和准确性。
用python写一个自动手机拨号代码
可以的,以下是一个简单的Python代码示例:
```python
import time
import subprocess
phone_number = "1234567890" # 手机号码
duration = 10 # 拨号时长(秒)
# 执行拨号命令
subprocess.call(["adb", "shell", "service", "call", "phone", "1", "s", phone_number])
# 等待拨号完成
time.sleep(duration)
# 执行挂断命令
subprocess.call(["adb", "shell", "input", "keyevent", "6"])
```
这个代码使用了Android Debug Bridge(ADB)工具来执行拨号和挂断命令。你需要先连接你的手机到电脑上,并确保已经安装了ADB工具。如果你使用的是Windows系统,你还需要将ADB工具的路径添加到系统环境变量中。
当你运行这个代码时,它会自动拨打指定的手机号码,并在指定的时长后挂断电话。你可以根据自己的需要修改代码中的参数。
阅读全文
相关推荐
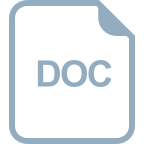
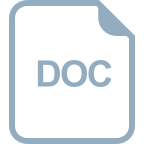
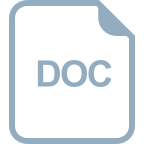
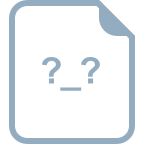

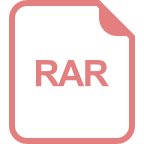
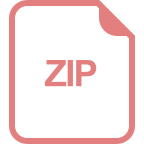
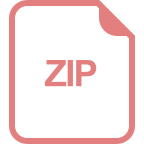
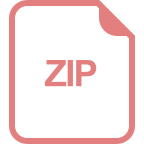
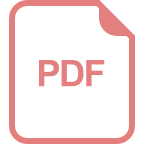
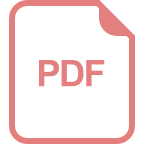
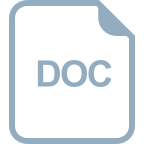
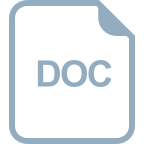


