SPRINGBOOT 下载
时间: 2023-11-03 16:59:50 浏览: 43
SPRINGBOOT通过以下三种方式实现文件下载功能:
1. 通过URL连接下载网络文件到本地:
```java
@RequestMapping("/netDownloadLocal")
public void downloadNet(String netAddress, String path) throws IOException {
URL url = new URL(netAddress);
URLConnection conn = url.openConnection();
InputStream inputStream = conn.getInputStream();
FileOutputStream fileOutputStream = new FileOutputStream(path);
int bytesum = 0;
int byteread;
byte[] buffer = new byte[1024];
while ((byteread = inputStream.read(buffer)) != -1) {
bytesum = byteread;
fileOutputStream.write(buffer, 0, byteread);
}
fileOutputStream.close();
}
```
2. 将文件的输入流循环写入响应输出流,实现文件下载:
```java
@RequestMapping("/downloadLocal")
public void downloadLocal(String path, HttpServletResponse response) throws IOException {
InputStream inputStream = new FileInputStream(path);
response.reset();
response.setContentType("application/octet-stream");
String filename = new File(path).getName();
response.addHeader("Content-Disposition", "attachment; filename=" + URLEncoder.encode(filename, "UTF-8"));
ServletOutputStream outputStream = response.getOutputStream();
byte[] b = new byte[1024];
int len;
while ((len = inputStream.read(b)) > 0) {
outputStream.write(b, 0, len);
}
inputStream.close();
}
```
3. 将网络文件获取到服务器后,经过服务器处理后响应给前端:
```java
@RequestMapping("/netDownLoadNet")
public void netDownLoadNet(String netAddress, String filename, boolean isOnLine, HttpServletResponse response) throws Exception {
URL url = new URL(netAddress);
URLConnection conn = url.openConnection();
InputStream inputStream = conn.getInputStream();
response.reset();
response.setContentType(conn.getContentType());
if (isOnLine) {
response.setHeader("Content-Disposition", "inline; filename=" + URLEncoder.encode(filename, "UTF-8"));
} else {
response.setHeader("Content-Disposition", "attachment; filename=" + URLEncoder.encode(filename, "UTF-8"));
}
byte[] buffer = new byte[1024];
int len;
OutputStream outputStream = response.getOutputStream();
while ((len = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, len);
}
inputStream.close();
}
```
相关推荐
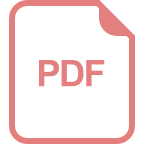
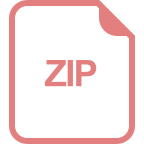
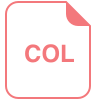
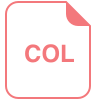
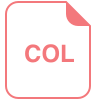
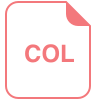
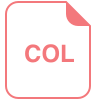









