c#打字练习软件设计
时间: 2023-12-05 08:40:01 浏览: 108
以下是一个简单的C#打字练习软件设计:
1. 首先,需要创建一个Windows窗体应用程序。
2. 在窗体中添加一个文本框和一个标签,用于显示练习文本和用户输入的文本。
3. 在窗体中添加一个计时器控件,用于计算用户完成练习所需的时间。
4. 在窗体中添加一个按钮,用于开始练习。
5. 在代码中,需要定义一个字符串数组,用于存储练习文本。
6. 在按钮的Click事件中,随机选择一个字符串数组中的文本,并将其显示在标签中。
7. 在文本框的TextChanged事件中,将用户输入的文本与练习文本进行比较,并将正确的字符显示为绿色,错误的字符显示为红色。
8. 在计时器的Tick事件中,更新计时器的显示,并检查用户是否完成了练习。
9. 如果用户完成了练习,停止计时器并显示完成时间和准确性。
以下是示例代码:
```csharp
public partial class Form1 : Form
{
private string[] texts = { "The quick brown fox jumps over the lazy dog.", "Sphinx of black quartz, judge my vow.", "How vexingly quick daft zebras jump!", "Pack my box with five dozen liquor jugs." };
private string currentText;
private int currentIndex;
private int correctCount;
private int errorCount;
private bool isStarted;
public Form1()
{
InitializeComponent();
}
private void btnStart_Click(object sender, EventArgs e)
{
if (!isStarted)
{
currentIndex = new Random().Next(texts.Length);
currentText = texts[currentIndex];
lblText.Text = currentText;
txtInput.Text = "";
correctCount = 0;
errorCount = 0;
lblAccuracy.Text = "";
lblTime.Text = "00:00:00";
timer1.Start();
isStarted = true;
}
}
private void txtInput_TextChanged(object sender, EventArgs e)
{
if (isStarted)
{
string inputText = txtInput.Text;
int minLength = Math.Min(currentText.Length, inputText.Length);
for (int i = 0; i < minLength; i++)
{
if (inputText[i] == currentText[i])
{
txtInput.Select(i, 1);
txtInput.SelectionColor = Color.Green;
}
else
{
txtInput.Select(i, 1);
txtInput.SelectionColor = Color.Red;
errorCount++;
}
}
if (inputText.Length > currentText.Length)
{
txtInput.Select(currentText.Length, inputText.Length - currentText.Length);
txtInput.SelectionColor = Color.Red;
errorCount += inputText.Length - currentText.Length;
}
lblAccuracy.Text = $"{100 * correctCount / (correctCount + errorCount)}%";
}
}
private void timer1_Tick(object sender, EventArgs e)
{
TimeSpan timeSpan = TimeSpan.FromSeconds(timer1.Interval / 1000 + timer1.Tag == null ? 0 : (int)timer1.Tag);
lblTime.Text = timeSpan.ToString("hh\\:mm\\:ss");
if (isStarted && txtInput.Text == currentText)
{
timer1.Stop();
MessageBox.Show($"Congratulations! You finished the text in {timeSpan.TotalSeconds} seconds with {lblAccuracy.Text} accuracy.");
isStarted = false;
}
}
}
```
阅读全文
相关推荐
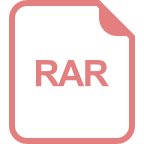
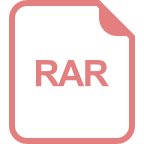
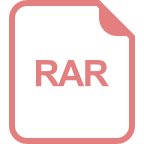
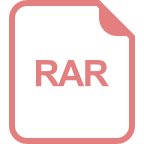
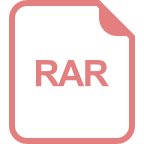
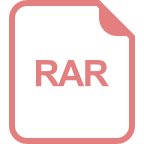
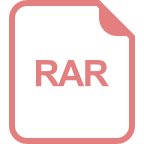
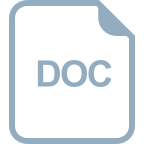
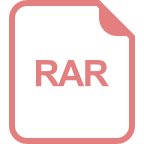
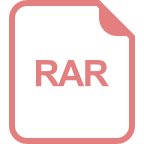
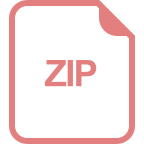