Python对记事本中的数字排序
时间: 2024-09-20 11:12:36 浏览: 37
Python本身并不直接支持从记事本文件(通常文本格式,如.txt)中读取数据并对其中的数字进行原地排序。但是,你可以通过以下步骤操作:
1. **读取内容**:首先,你需要使用内置的`open()`函数打开记事本文件,并使用`readlines()`方法读取所有行作为列表。
```python
with open('notebook.txt', 'r') as file:
lines = file.readlines()
```
2. **解析数字**:然后遍历每一行,将字符串转换成整数或浮点数。可以使用`int()`或`float()`函数。这一步需要处理可能出现的错误,比如非数字字符。
```python
numbers = [int(line) for line in lines if line.strip().isdigit()]
```
3. **排序**:最后,你可以使用`sorted()`函数对数字列表进行排序。如果想保持原始位置不变,可以先保存原始索引,再根据新的排序顺序重构。
```python
sorted_numbers, indices = zip(*sorted(zip(numbers, range(len(numbers)))))
```
4. **写回文件**:如果你想把排序后的数字替换回原来的文件,可以用`enumerate()`结合`line_number`来生成新内容。
```python
new_content = ['{}{}'.format(num, '\n' if i != len(sorted_numbers)-1 else '') for i, num in enumerate(sorted_numbers)]
with open('notebook.txt', 'w') as file:
file.writelines(new_content)
```
阅读全文
相关推荐
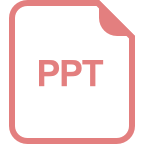
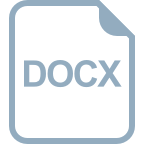
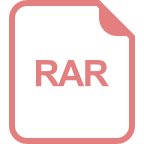

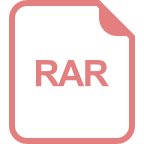
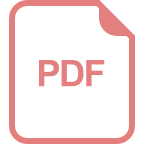
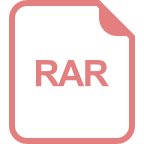
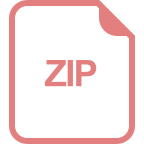
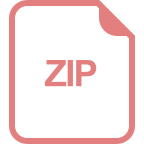
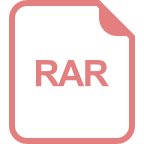
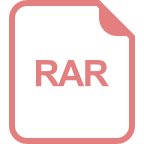
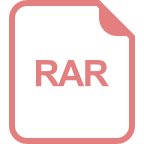
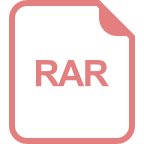
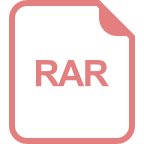
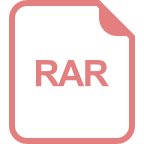