python pyqt6把每一张图片持续输出到画布上
时间: 2024-09-26 09:03:44 浏览: 30
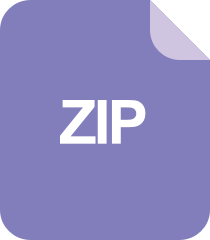
Python绘图画板程序 自由绘制 直线 矩形 椭圆 画笔调整 PyQt6
在Python中使用PyQt6动态地将图片持续更新到画布(通常是QLabel或QPixmap)上,可以利用定时器或者事件驱动的方式实现。这里有个简单示例,假设你有一个图片列表,会每隔一段时间更换当前显示的图片:
```python
import sys
from PyQt6.QtCore import QTimer, Qt
from PyQt6.QtGui import QPixmap
from PyQt6.QtWidgets import QLabel, QApplication
class ImageUpdater(QTimer):
def __init__(self, parent=None):
super().__init__(parent)
self.image_list = ['image1.jpg', 'image2.jpg', 'image3.jpg'] # 图片文件路径
self.current_index = 0
self.label = QLabel(parent)
self.update_timer()
def update_image(self):
pixmap = QPixmap(self.image_list[self.current_index])
if pixmap.isNull():
print(f"Error loading image {self.image_list[self.current_index]}")
return
self.label.setPixmap(pixmap)
self.current_index = (self.current_index + 1) % len(self.image_list) # 循环显示
def update_timer(self):
self.timeout.connect(self.update_image)
self.start(5000) # 每隔5秒更新一次
def main():
app = QApplication(sys.argv)
window = QLabel("Image Updater", parent=None)
updater = ImageUpdater(window)
window.show()
sys.exit(app.exec())
if __name__ == "__main__":
main()
```
在这个示例中,我们创建了一个`ImageUpdater`类,它继承自`QTimer`,并将图片列表作为成员变量。`update_image`方法负责加载和设置新的图像,并更新索引以便循环显示。`update_timer`方法启动定时器并在其回调中触发`update_image`。
注意,你需要替换`image1.jpg`, `image2.jpg`, 和`image3.jpg`为实际图片文件路径。
阅读全文
相关推荐
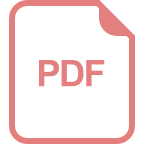
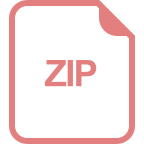
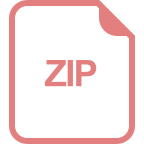
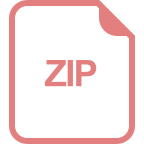
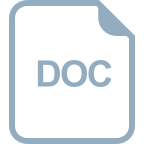
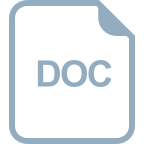


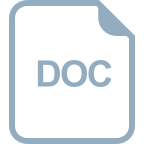







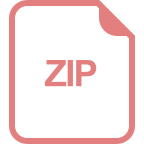