如上 整体如何实现 复制粘贴就能使用的版本
时间: 2024-09-30 13:03:52 浏览: 17
要实现整体的离线添加客户功能并能复制粘贴数据,首先你需要一个基础的HTML模板和一些JavaScript来处理输入、本地存储以及表单验证。这是一个简化版的示例,你可以直接复制粘贴到项目中调整:
**HTML部分:**
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>离线客户管理</title>
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
<script src="https://unpkg.com/vue@2"></script>
<script src="https://cdn.jsdelivr.net/npm/vuex@3"></script>
</head>
<body>
<div id="app">
<form @submit.prevent="addCustomer">
<el-input v-model="newCustomer.name" placeholder="客户名称"></el-input>
<el-input v-model="newCustomer.contact" placeholder="客户联系方式"></el-input>
<!-- 其他输入字段... -->
<button type="submit">添加客户</button>
</form>
<ul>
<li v-for="(customer, index) in customers" :key="index">
<span>{{ customer.name }}</span>
<span>{{ customer.contact }}</span>
<!-- 删除按钮 -->
<button @click="deleteCustomer(index)">删除</button>
</li>
</ul>
</div>
<script src="app.js"></script>
</body>
</html>
```
**JavaScript部分 (app.js)**:
```javascript
// store.js (放在单独的js文件)
const store = new Vuex.Store({
state: {
customers: [], // 假设已存在本地存储
newCustomer: {} // 新客户数据
},
mutations: {
setNewCustomer(state, customer) {
state.newCustomer = customer;
},
addCustomer(state) {
state.customers.push(state.newCustomer);
localStorage.setItem('customers', JSON.stringify(state.customers));
state.newCustomer = {}; // 清空新客户数据
},
deleteCustomer(state, index) {
state.customers.splice(index, 1);
localStorage.setItem('customers', JSON.stringify(state.customers));
}
},
actions: {
loadCustomers({ commit }) {
const storedCustomers = localStorage.getItem('customers');
if (storedCustomers) {
commit('setCustomers', JSON.parse(storedCustomers));
}
}
},
getters: {
getCustomers(state) {
return state.customers;
}
}
});
new Vue({
el: '#app',
store,
data() {
return {
newCustomer: {}
};
},
computed: {
customers() {
return this.$store.getters.getCustomers;
}
},
methods: {
addCustomer() {
// 进行必要的表单验证,然后使用 mutation 添加到store
// 可能包含错误处理、数据清洗等逻辑
this.$store.commit('addCustomer', this.newCustomer);
},
deleteCustomer(index) {
this.$store.commit('deleteCustomer', index);
},
init顾客() {
this.$store.dispatch('loadCustomers');
}
},
created() {
this.initCustomers();
}
});
```
这个版本仅支持基本的添加和删除,如果需要更复杂的验证、导入导出数据等功能,可能需要额外的代码来处理。记得替换掉`localStorage`部分为适合你的离线存储方案,比如IndexedDB。
相关推荐
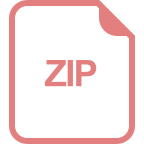
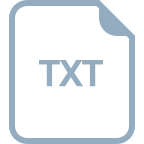















