设计并实现求子串的算法,利用C语言
时间: 2024-10-10 09:04:04 浏览: 38
在C语言中,我们可以使用动态规划(Dynamic Programming)的方法来设计一个高效的求解子串问题的算法,例如著名的KMP(Knuth-Morris-Pratt)算法或Boyer-Moore算法。这里以KMP算法为例,它用于在一个主字符串中查找指定的模式串:
```c
#include <stdio.h>
#include <string.h>
// 创建next数组存储模式串的失败跳转表
void computeNext(char pattern[], int next[]) {
int len = strlen(pattern);
next[0] = -1;
int j = -1;
for (int i = 1; i < len; ++i) {
while (j != -1 && pattern[j + 1] != pattern[i]) {
j = next[j];
}
if (pattern[j + 1] == pattern[i]) {
j++;
}
next[i] = j;
}
}
// KMP匹配函数
bool kmpMatch(char text[], char pattern[]) {
int lenText = strlen(text);
int lenPattern = strlen(pattern);
computeNext(pattern, next);
int j = 0;
for (int i = 0; i < lenText; ++i) {
while (j != -1 && text[i] != pattern[j + 1]) {
j = next[j];
}
if (text[i] == pattern[j + 1]) {
j++;
}
// 如果找到模式串,返回true;若遍历完主串还没找到,则模式串不存在
if (j == lenPattern) {
return true;
}
}
return false;
}
int main() {
char text[] = "Hello, how are you?";
char pattern[] = "how";
if (kmpMatch(text, pattern)) {
printf("Substring found: %s\n", pattern);
} else {
printf("Substring not found.\n");
}
return 0;
}
```
在这个例子中,`computeNext`函数计算了模式串的失败跳转表,而`kmpMatch`函数则使用这个表在主字符串中搜索模式串。如果找到匹配,`main`函数会打印出子串。
阅读全文
相关推荐
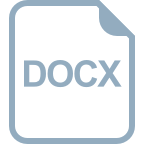
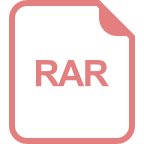
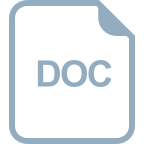



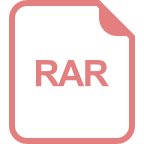
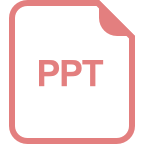
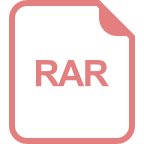
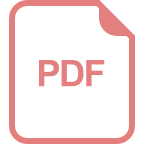
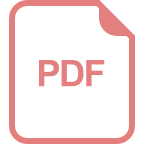
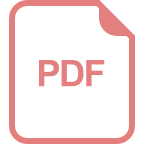
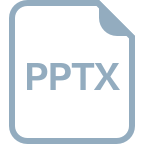
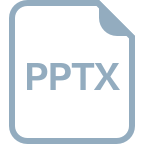
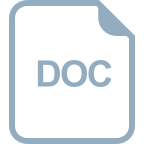
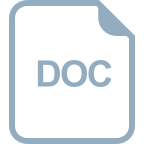