给定一串十进制数字,共n位,要求将这些数字分成m段,由此得到了m个整数。如何划分使得m个整数的乘积最大?c语言实现
时间: 2024-10-07 10:03:23 浏览: 60
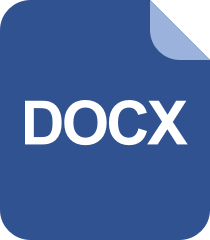
串口十进制输出.docx
要解决这个问题,我们可以使用一种叫做“分治”的策略,以及一些数学技巧来确定最佳分割点。首先,你需要对给定的数字进行排序,然后考虑每次从左到右切分,直到只剩下一个元素为止。每次切分时,比较当前连续部分的乘积与下一个连续部分的乘积,选择其中更大的作为分割点。
下面是一个简单的C语言实现,假设输入的数字数组名为`nums`,长度为`n`:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 对数组进行排序
void sort(int arr[], int n) {
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
// 计算连续部分的乘积
long long product(int start, int end, int nums[]) {
long long result = 1;
for (int i = start; i <= end; ++i) {
result *= nums[i];
}
return result;
}
// 分割数组并求解最大乘积
long long maxProduct(int* nums, int n, int m) {
if (m == 1 || n == 1) return nums[0]; // 基线条件:单个元素或只有一个分割
sort(nums, n); // 先排序
int len = n / m; // 每个部分的预期长度
long long bestProduct = 0;
for (int i = 0; i < len; ++i) { // 从头开始切分
bestProduct = max(bestProduct, product(i, i + len - 1, nums));
if (i + len >= n) break; // 当前部分已覆盖数组结尾
}
return bestProduct;
}
int main() {
int nums[] = {3, 4, 6, 5, 7};
int n = sizeof(nums) / sizeof(nums[0]);
int m = 3; // 要把数字分成3段
printf("最大乘积: %lld\n", maxProduct(nums, n, m));
return 0;
}
```
这段代码首先对数组进行排序,然后依次检查不同的切割位置,寻找最大的乘积。注意,这个实现假设了`m`能整除`n`;如果不能,则可能需要调整算法以处理剩余的部分。
阅读全文
相关推荐
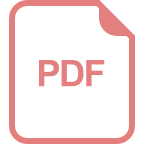
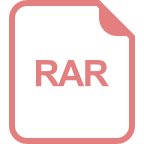
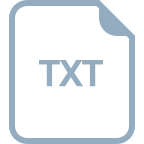
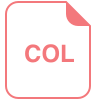
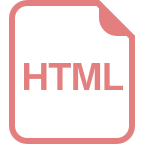
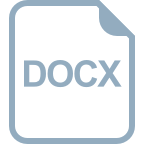