判断字符串“A+B”中+前后是否一致 C语言
时间: 2024-10-08 13:16:26 浏览: 15
在C语言中,判断字符串"A+B"中"+"前后是否一致可以通过遍历字符串并比较字符来实现。假设我们有两个字符串A和B,我们需要先将它们连接起来形成新的字符串str = A + B,然后检查str是否满足条件:所有 "+" 符号前面都是字符,即非"+"字符紧跟着"+"字符。
以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <stdbool.h>
#include <string.h>
bool check_plus_sign(const char *str) {
int len = strlen(str);
bool previous_is_plus = false;
for (int i = 0; i < len; ++i) {
if (str[i] == '+') {
if (previous_is_plus) {
return false; // 如果连续两个加号,返回false
}
previous_is_plus = true;
} else {
if (!previous_is_plus) {
return false; // 如果不是加号后跟字符,返回false
}
previous_is_plus = false;
}
}
// 检查最后一个 '+' 后是否有字符
if (previous_is_plus) {
return false;
}
return true; // 所有条件都满足,返回true
}
int main() {
const char *A = "abc";
const char *B = "def+ghi";
char str[] = A + B; // 连接两个字符串
if (check_plus_sign(str)) {
printf("字符串 '+' 前后一致\n");
} else {
printf("字符串 '+' 前后不一致\n");
}
return 0;
}
```
在这个例子中,`check_plus_sign`函数会检查字符串是否满足要求,如果满足则返回`true`,否则返回`false`。注意,这个示例假设输入的A和B以及连接后的结果都在当前栈空间内,实际应用中可能需要考虑内存管理等问题。
阅读全文
相关推荐
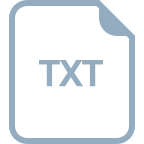
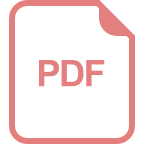
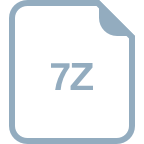
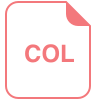
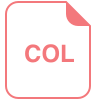
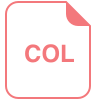
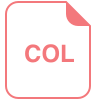
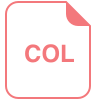
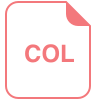
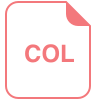
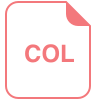
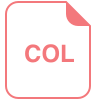
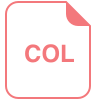
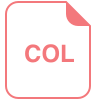
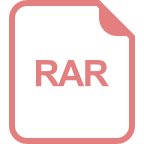
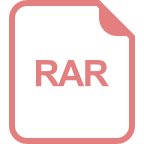