在Java中进行复数的加减乘除实验原理
时间: 2024-11-28 21:27:13 浏览: 0
在Java中进行复数的加减乘除操作,需要利用自定义类来表示复数,并提供相应的运算方法。首先,我们可以创建一个名为`ComplexNumber`的类,包含两个成员变量:实部(`real`)和虚部(`imaginary`),分别代表复数的a和b。然后,可以定义四个方法:
1. `add(ComplexNumber other)`:用于两个复数相加,通过将各自实部和虚部分别相加得到结果。
2. `subtract(ComplexNumber other)`:用于两个复数相减,通过将各自实部相减、虚部相减得到结果。
3. `multiply(ComplexNumber other)`:用于两个复数相乘,计算(a + bi) * (c + di) = (ac - bd) + (bc + ad)i。
4. `divide(ComplexNumber other)`:用于两个非零复数相除,计算(a + bi) / (c + di) = [(ac + bd) / (c^2 + d^2), (-bc + ad) / (c^2 + d^2)],确保分母不为0。
在类中,可以使用封装和数据隐藏的原则,只暴露必要的构造方法和上述运算方法,保持类的内部状态私有。以下是简化的代码示例:
```java
public class ComplexNumber {
private double real;
private double imaginary;
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 运算方法
public ComplexNumber add(ComplexNumber other) {
return new ComplexNumber(this.real + other.real, this.imaginary + other.imaginary);
}
public ComplexNumber subtract(ComplexNumber other) {
return new ComplexNumber(this.real - other.real, this.imaginary - other.imaginary);
}
public ComplexNumber multiply(ComplexNumber other) {
double realPart = this.real * other.real - this.imaginary * other.imaginary;
double imagPart = this.real * other.imaginary + this.imaginary * other.real;
return new ComplexNumber(realPart, imagPart);
}
public ComplexNumber divide(ComplexNumber other) {
if (other.real == 0 && other.imaginary == 0) throw new ArithmeticException("Cannot divide by zero");
double denominator = other.real * other.real + other.imaginary * other.imaginary;
double realPart = (this.real * other.real + this.imaginary * other.imaginary) / denominator;
double imagPart = (this.imaginary * other.real - this.real * other.imaginary) / denominator;
return new ComplexNumber(realPart, imagPart);
}
// 获取和设置方法,省略...
}
```
阅读全文
相关推荐
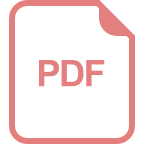
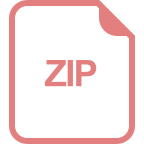
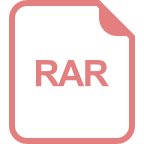
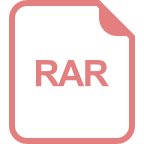
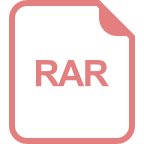
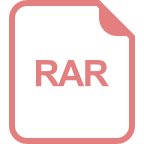
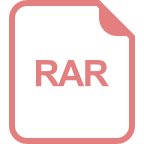
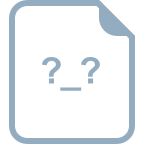
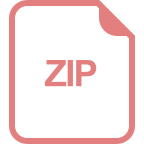
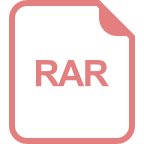
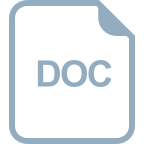
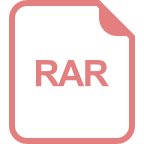
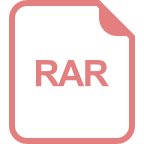