在c语言中建立带表头节点的链表L,满足用户从键盘正序位输入数据元素
时间: 2024-09-29 19:13:01 浏览: 50
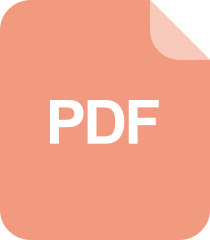
C语言进阶-第五讲 数据结构与链表
在C语言中创建一个带表头节点的链表L,允许用户按顺序从键盘输入数据元素,你可以按照以下步骤操作:
1. **定义结构体**:
首先,创建一个包含数据域和指针域的结构体表示链表节点。例如:
```c
struct Node {
int data; // 数据域存储输入的数据
struct Node* next; // 指向下一个节点的指针
};
```
2. **定义全局变量**:
初始化一个指向链表头部的指针 `head` 作为空指针,表示链表刚开始是空的。
```c
struct Node* head = NULL;
```
3. **输入数据函数**:
创建一个函数用于获取用户输入并插入新节点到链表中。这个函数应该循环接收输入直到用户停止输入特定标志(如回车两次)。
```c
void insertData() {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode == NULL) {
printf("内存分配失败!\n");
return;
}
newNode->data = -1; // 空节点暂时标记,后续处理删除
scanf("%d", &newNode->data); // 输入数据
newNode->next = head;
head = newNode;
while (getchar() != '\n' && getchar() != EOF) { // 接收更多输入,直到换行符或文件结束
newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode == NULL) {
printf("内存分配失败!\n");
break;
}
newNode->data = getchar() - '0'; // 将字符转换为整数
newNode->next = head;
head = newNode;
}
}
```
4. **链表遍历**:
如果需要打印链表内容,可以编写一个函数遍历整个链表。
```c
void printList() {
struct Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
```
5. **主程序**:
在主函数中调用上述函数,并提供交互界面让用户输入数据。
```c
int main() {
while (true) {
insertData();
printList(); // 打印链表,然后检查是否继续
printf("是否继续? (y/n): ");
char choice = getchar();
if (choice == 'n' || choice == 'N') {
break;
}
}
return 0;
}
```
阅读全文
相关推荐
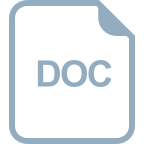
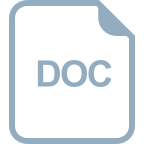
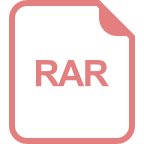
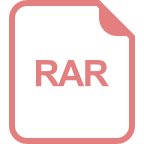
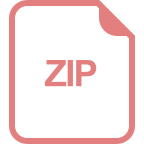
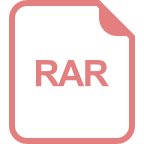
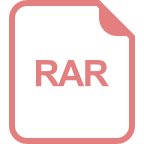
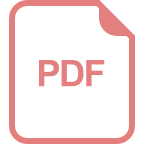
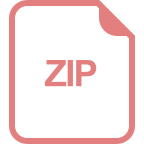
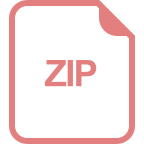
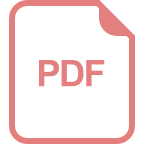
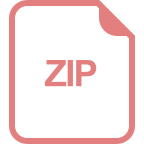
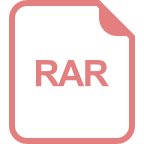
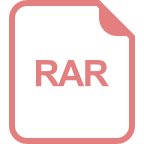
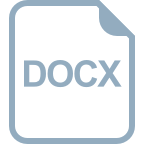