JSON字符串如何正确地转换为Java Date对象?
时间: 2024-10-24 21:11:59 浏览: 90
要将JSON字符串正确转换为Java的Date对象,你需要按照Java的日期时间API规范(如`java.util.Calendar`、`java.time.LocalDate`或`java.time.LocalDateTime`),尤其是如果你的日期字符串符合ISO 8601标准(YYYY-MM-DD或YYYY-MM-dd'T'HH:mm:ss.SSSZ)。以下是基本步骤:
1. **使用`SimpleDateFormat`或`DateTimeFormatter`**:
- 对于`SimpleDateFormat`,你可以创建一个实例并指定合适的日期格式,然后使用`parse()`方法:
```java
String dateString = "2000-03-02";
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
Date date = formatter.parse(dateString);
```
2. **使用`java.time`包**:
- 使用`LocalDate`和`DateTimeFormatter`,例如:
```java
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy年MM月dd日");
LocalDate localDate = LocalDate.parse(dateString, formatter);
// 如果需要带时分秒,可以改为LocalDateTime
LocalDateTime dateTime = LocalDateTime.parse(dateString, formatter.with(TemporalAdjusters.ofTime ZoneId.systemDefault()));
```
3. **异常处理**:
转换过程中可能会抛出`ParseException`,因此通常建议捕获并处理它。
4. **`Instant`与`ZonedDateTime`**:
如果日期带有时区,可以考虑使用`Instant`和`ZonedDateTime`:
```java
String dateStringWithTimeZone = "2000-03-02T08:00:00+08:00";
ZonedDateTime zonedDateTime = ZonedDateTime.parse(dateStringWithTimeZone);
```
记得检查输入日期是否有效,如果不确定,可以在转换前添加校验。
阅读全文
相关推荐








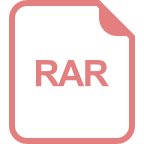









