数据库课程设计JavaSpring
时间: 2024-12-28 10:25:08 浏览: 5
### Java Spring 数据库课程设计示例教程
#### 设计概述
数据库课程设计提供了深入了解数据库原理和实际操作的机会。通过结合Java和Spring框架,可以构建功能强大的应用程序来管理数据[^1]。
#### 技术栈介绍
- **编程语言**: Java
- **Web框架**: Spring Boot
- **持久层技术**: JPA (Java Persistence API)
- **数据库管理系统**: MySQL
#### 创建项目结构
首先,在IDE中创建一个新的Spring Boot项目,并配置`pom.xml`文件引入必要的依赖项:
```xml
<dependencies>
<!-- Spring Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- MySQL Connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
```
#### 配置数据库连接
编辑`application.properties`文件设置MySQL数据库的连接参数:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/student?useSSL=false&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=password
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL8Dialect
```
#### 定义实体类
定义一个名为`StudentInfo`的实体类映射到之前提到的学生信息表[^3]:
```java
@Entity
@Table(name = "studentinfo")
public class StudentInfo {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private int age;
private String major;
// Getters and Setters...
}
```
#### 编写Repository接口
利用Spring Data JPA简化CRUD操作,只需声明继承自`JpaRepository`的接口即可自动获得常用方法的支持:
```java
@Repository
public interface StudentInfoRepository extends JpaRepository<StudentInfo, Long> {}
```
#### 控制器实现业务逻辑
编写RESTful风格的服务端控制器处理HTTP请求并返回JSON响应给前端应用:
```java
@RestController
@RequestMapping("/api/students")
public class StudentController {
@Autowired
private StudentInfoRepository studentRepo;
@GetMapping("")
public List<StudentInfo> getAllStudents() {
return studentRepo.findAll();
}
@PostMapping("")
public StudentInfo createNewStudent(@RequestBody StudentInfo newStudent) {
return studentRepo.save(newStudent);
}
}
```
以上就是一个完整的基于Java与Spring框架下的小型数据库课程设计方案实例说明。此方案不仅涵盖了基础的数据访问模式,还展示了如何快速搭建一套具有增删改查能力的应用程序原型。
阅读全文
相关推荐



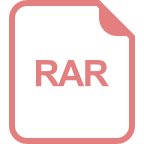












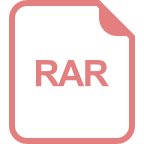