写一个post请求,有以下要求: 请求地址 URL:http://172.16.10.13:18080/api/sso/getUserInfo post请求,Body格式:form-data header中的key有这些参数:timestamp,appKey,sign,,version body有这些参数:authCode,account,nickName,name 这个请求的返回示例为{ "success": true, "code": 200, "message": "请求成功", "data": { "account": "50011737", "nickName": "张三", "name": "张三" } } 将account解析出来,用Java代码写
时间: 2023-08-11 20:07:10 浏览: 221
好的,以下是使用Java代码发送post请求并解析返回数据中的account字段的示例代码:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
String url = "http://172.16.10.13:18080/api/sso/getUserInfo";
Map<String, String> headers = new HashMap<>();
headers.put("timestamp", "your_timestamp");
headers.put("appKey", "your_appKey");
headers.put("sign", "your_sign");
headers.put("version", "your_version");
Map<String, String> body = new HashMap<>();
body.put("authCode", "your_authCode");
body.put("account", "your_account");
body.put("nickName", "your_nickName");
body.put("name", "your_name");
try {
String response = sendPostRequest(url, headers, body);
String account = parseAccountFromResponse(response);
System.out.println("Account: " + account);
} catch (IOException e) {
e.printStackTrace();
}
}
private static String sendPostRequest(String url, Map<String, String> headers, Map<String, String> body) throws IOException {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
// Set request headers
for (Map.Entry<String, String> entry : headers.entrySet()) {
con.setRequestProperty(entry.getKey(), entry.getValue());
}
StringBuilder postData = new StringBuilder();
// Set request body
for (Map.Entry<String, String> entry : body.entrySet()) {
if (postData.length() != 0) {
postData.append("&");
}
postData.append(entry.getKey()).append("=").append(entry.getValue());
}
byte[] postDataBytes = postData.toString().getBytes("UTF-8");
con.setDoOutput(true);
con.getOutputStream().write(postDataBytes);
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
private static String parseAccountFromResponse(String response) {
// Parse the account field from the response JSON
// Here is a simple example assuming the response is always in the same format as the provided example
int startIndex = response.indexOf("\"account\": \"") + "\"account\": \"".length();
int endIndex = response.indexOf("\"", startIndex);
return response.substring(startIndex, endIndex);
}
}
```
请将代码中的 `your_` 替换为实际的值,并根据实际情况调整解析返回数据中account字段的方法`parseAccountFromResponse`,以适应实际返回数据的格式。
阅读全文
相关推荐
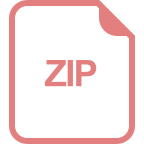















