python和MySQL数据库怎么连接
时间: 2024-11-05 18:02:19 浏览: 18
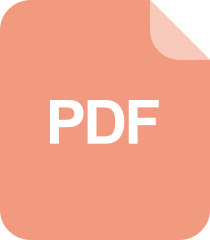
python,mysql连接.pdf
在Python中,可以使用`mysql-connector-python`库或`pymysql`库来连接MySQL数据库。以下是基本步骤:
1. **安装库**:
- 安装`mysql-connector-python`:
```bash
pip install mysql-connector-python
```
或者安装`pymysql`:
```bash
pip install pymysql
```
2. **连接示例**(使用`mysql-connector-python`):
```python
import mysql.connector
# 创建连接
cnx = mysql.connector.connect(
host="your_host",
user="your_username",
password="your_password",
database="your_database"
)
# 打开游标
cursor = cnx.cursor()
# 执行SQL查询或其他操作
query = "SELECT * FROM your_table"
cursor.execute(query)
# 获取结果
rows = cursor.fetchall()
for row in rows:
print(row)
# 关闭游标和连接
cursor.close()
cnx.close()
```
3. **连接示例**(使用`pymysql`):
```python
import pymysql
# 连接数据库
db = pymysql.connect(host="your_host", user="your_username", password="your_password", db="your_database")
try:
# 创建游标
cursor = db.cursor()
# 执行查询
query = "SELECT * FROM your_table"
cursor.execute(query)
# 获取数据并打印
results = cursor.fetchall()
for row in results:
print(row)
finally:
# 关闭游标和连接
cursor.close()
db.close()
```
阅读全文
相关推荐
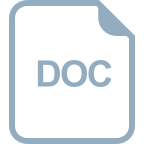
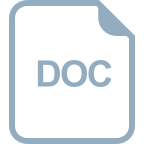
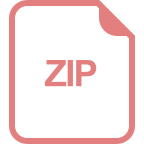
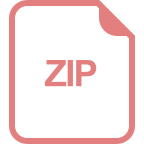
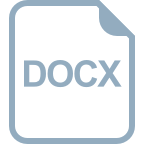
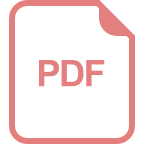
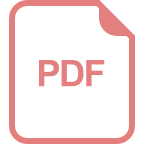
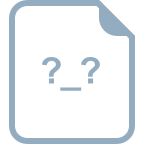
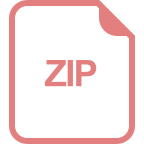
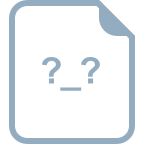
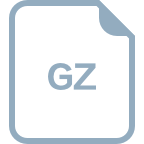
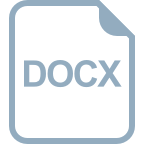
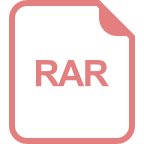
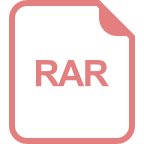