java编写一个程序,完成从某账户取款和存款的操作。(1)输人存取金额时,如果非数值型,捕获异常并进行处理(系统异常)。(2)操作账户类时,如果取款数大于金额则作为异常处理(自定义异常)。
时间: 2024-10-19 07:17:21 浏览: 45
在Java中,你可以创建一个`BankAccount`类,它包含一个余额字段和两个方法:`deposit`用于存款,`withdraw`用于取款。为了满足题目中的要求,我们将使用`try-catch`块捕获输入错误和取款超限的异常。首先,我们需要自定义一个`InvalidInputException`异常用于处理非数值输入,以及一个`InsufficientBalanceException`异常用于取款超限。
```java
import java.text.ParseException;
import java.util.Scanner;
class InvalidInputException extends Exception {
public InvalidInputException(String message) {
super(message);
}
}
class InsufficientBalanceException extends Exception {
public InsufficientBalanceException(double amount) {
this.message = "Insufficient balance! Cannot withdraw more than current balance.";
}
}
class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
// 存款方法
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
System.out.println("Deposit successful. New balance: " + balance);
} else {
throw new InvalidInputException("Deposit amount must be a positive number.");
}
}
// 取款方法
public void withdraw(double amount) throws InsufficientBalanceException {
if (amount > 0 && amount <= balance) {
balance -= amount;
System.out.println("Withdrawal successful. New balance: " + balance);
} else if (amount <= 0) {
throw new InvalidInputException("Withdrawal amount must be a positive number.");
} else {
throw new InsufficientBalanceException(amount);
}
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
try {
System.out.println("Enter your initial account balance:");
BankAccount account = new BankAccount(Double.parseDouble(scanner.nextLine()));
while (true) {
System.out.println("\n1. Deposit\n2. Withdraw\n3. Exit");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.print("Enter the deposit amount: ");
account.deposit(scanner.nextDouble());
break;
case 2:
System.out.print("Enter the withdrawal amount: ");
account.withdraw(scanner.nextDouble());
break;
case 3:
System.exit(0);
break;
default:
System.out.println("Invalid option. Please try again.");
}
}
} catch (NumberFormatException e) {
System.out.println("Invalid input! Please enter a numeric value.");
} catch (InvalidInputException | InsufficientBalanceException e) {
System.out.println(e.getMessage());
} finally {
scanner.close();
}
}
}
```
在这个程序中,用户可以选择存款或取款,系统会检查输入是否合法并处理可能出现的异常情况。当用户选择退出时,程序结束。注意,这里假设用户能够提供正确的初始存款金额。实际应用中,可能需要更完整的用户输入验证。
阅读全文
相关推荐







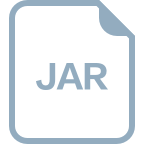
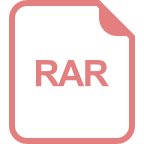




