用java写一个scheduled 定时脚本,要求时间格式为每天下午4点触发一次时间,这个时间来源是动态的,从配置文件配置的
时间: 2024-10-28 09:10:26 浏览: 6
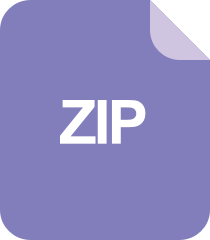
cron-last-sunday:在每月的第一个,第n个或最后一个工作日运行cron任务
在Java中,我们可以使用`ScheduledExecutorService`和`TimerTask`来编写定时任务。如果时间是从配置文件动态获取的,你可以通过读取配置信息并将其转换为`java.util.concurrent.TimeUnit`和`long`形式,代表定时的时间间隔。这里是一个简单的示例:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.time.LocalTime;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class DynamicScheduledJob {
private ScheduledExecutorService executor;
public void start() {
// 从配置文件读取时间和频率
String configFilePath = "config.properties"; // 配置文件路径
LocalTime afternoonFour = getLocalTimeFromConfig(configFilePath, "schedule.hour", "schedule.minute");
// 创建一个ScheduledExecutorService
executor = Executors.newSingleThreadScheduledExecutor();
// 设置定时任务,每天下午4点执行
Runnable job = () -> {
System.out.println("Task executed at " + afternoonFour);
// 这里可以替换为你要执行的具体业务逻辑
};
// 使用cron表达式表示每天下午4点
String cronPattern = "0 16 * * ?";
executor.scheduleAtFixedRate(job, afternoonFour.toSecondOfDay(), 1, TimeUnit.DAYS); // 每天执行一次
try {
// 启动线程并等待
executor.awaitTermination(Long.MAX_VALUE, TimeUnit.NANOSECONDS);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
private LocalTime getLocalTimeFromConfig(String filePath, String hourKey, String minuteKey) {
int hour, minute;
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
if (line.contains(hourKey)) {
hour = Integer.parseInt(line.split("=")[1]);
} else if (line.contains(minuteKey)) {
minute = Integer.parseInt(line.split("=")[1]);
}
}
return LocalTime.of(hour, minute);
} catch (IOException e) {
throw new RuntimeException("Failed to read time from config file", e);
}
}
// 停止定时任务
public void stop() {
if (executor != null) {
executor.shutdownNow();
}
}
}
```
在这个例子中,你需要有一个`config.properties`文件,格式如下:
```
schedule.hour=16
schedule.minute=0
```
然后创建`DynamicScheduledJob`实例,调用`start()`开始定时任务,并在需要的时候调用`stop()`停止任务。
阅读全文
相关推荐
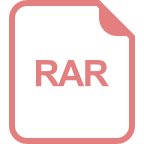
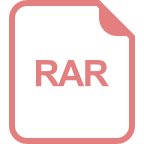
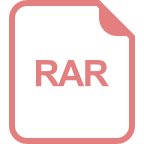
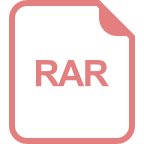
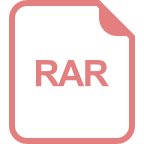
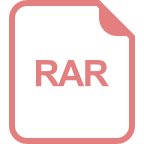
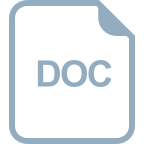
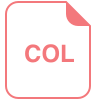
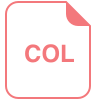
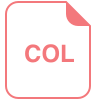
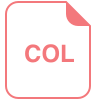
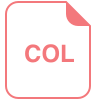
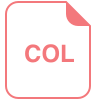
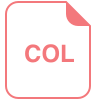
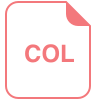
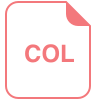

