linux进阶练习题
时间: 2024-12-26 17:17:23 浏览: 6
### 高级 Linux 练习题及答案
#### 进程与线程编程挑战
对于希望深入理解Linux进程和线程机制的人来说,下面是一些具有代表性的练习题:
1. **创建守护进程**
编写一段C代码来创建一个守护进程,在后台持续记录系统的当前时间到指定的日志文件中。该程序应该能够处理SIGTERM信号并优雅退出。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#include <sys/types.h>
#include <fcntl.h>
void sigterm_handler(int signum){
printf("Received SIGTERM\n");
exit(0);
}
int main(){
pid_t pid;
/* Fork off the parent process */
pid = fork();
if (pid < 0) {
fprintf(stderr, "fork failed!\n");
exit(EXIT_FAILURE);
}
// We got a good PID, now detach from terminal.
if(pid > 0){
exit(EXIT_SUCCESS);
}
umask(0);
// Create new SID for child process
sid = setsid();
if(sid < 0){
exit(EXIT_FAILURE);
}
close(STDIN_FILENO);
close(STDOUT_FILENO);
close(STDERR_FILENO);
signal(SIGCHLD,SIG_IGN);
signal(SIGHUP,SIG_IGN);
signal(SIGTERM,sigterm_handler);
while(true){
FILE *fp=fopen("/var/log/mydaemon.log","a+");
time_t rawtime;
struct tm *info;
char buffer[80];
time(&rawtime);
info=localtime(&rawtime);
strftime(buffer,sizeof(buffer),"%Y-%m-%d %H:%M:%S",info);
fprintf(fp,"%s\n",buffer);
fclose(fp);
sleep(60);
}
}
```
2. **多线程矩阵乘法**
编写一个多线程应用程序执行两个大尺寸浮点数矩阵相乘操作。每个工作线程负责计算结果矩阵的一行或多行。确保正确同步访问共享资源以防止竞争条件的发生[^1]。
```cpp
#include <iostream>
#include <pthread.h>
using namespace std;
#define N 4 // Matrix size
float A[N][N], B[N][N], C[N][N];
struct v { int row; }; // Used as argument to thread function
// Function that will be executed by each thread
void* multiply(void* arg){
struct v *data=(struct v*)arg;
int sum=0;
for(int i=0;i<N;++i)
for(int j=0;j<N;++j)
C[data->row][j]+=A[data->row][i]*B[i][j];
pthread_exit(NULL);
}
int main(){
srand(time(NULL));
pthread_t threads[N];
struct v vec[N];
// Initialize matrices with random values between 0 and 9
for(int i=0;i<N;++i)
for(int j=0;j<N;++j){
A[i][j]=rand()%10;
B[i][j]=rand()%10;
C[i][j]=0;
}
// Create one thread per matrix row
for(long t=0;t<N;++t){
vec[t].row=t;
pthread_create(&threads[t],NULL,multiply,(void*)&vec[t]);
}
// Wait until all threads have completed their work
for(long t=0;t<N;++t++)
pthread_join(threads[t], NULL);
cout << "\nResultant Matrix:\n";
for(int i=0;i<N;++i){
for(int j=0;j<N;++j)
cout<<C[i][j]<<" ";
cout<<"\n";
}
return 0;
}
```
阅读全文
相关推荐










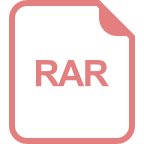








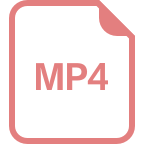