fortran将循环写入一个字符串
时间: 2024-09-09 17:13:12 浏览: 86
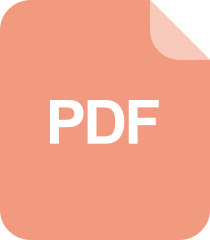
学习Fortran语言的20个注意事项及其基本案例
Fortran是一种古老的编程语言,主要用于数值计算和科学工程应用。在Fortran中,如果你想要把循环结构转换成字符串并存储起来,通常会借助于字符串连接或I/O函数。以下是一个简单的例子,展示了如何用Fortran编写一个循环并将它的迭代次数作为字符串输出:
```fortran
integer :: i, n, str_len
character(len=50) :: str
n = 10 ! 假设我们要进行10次循环
str_len = len(str)
do i = 1, n
str = trim(str)//trim(adjustl(char(i))) ! 使用//进行字符串连接,trim去除前后的空格
if (i < n) then
str = str // " times, "
else
str = str // " times."
end if
end do
write(*, *) 'The loop will be executed ', str
```
在这个例子中,我们创建了一个字符变量`str`,然后在每次循环中增加相应的迭代次数,并通过`//`操作符将其追加到原有字符串上。最后,使用`write`函数将结果打印出来。
阅读全文
相关推荐
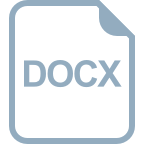
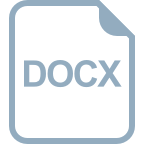
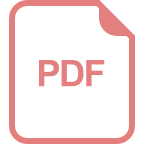
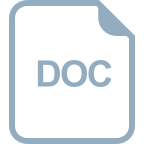
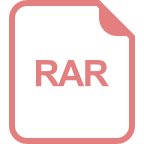
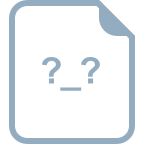
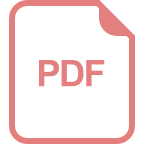
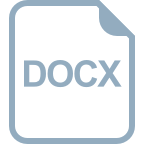
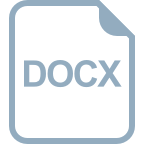
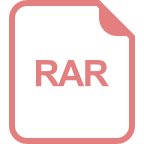
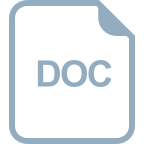
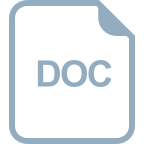
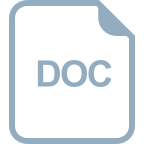
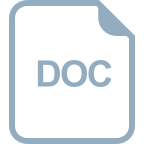
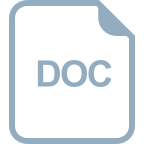
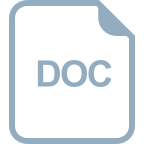
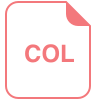
