使用jdbctemplate + spring+sprinfMvc简单实现学生表student的增删改查
时间: 2023-12-20 14:05:36 浏览: 63
使用JdbcTemplate结合Spring和Spring MVC可以更加方便地实现学生表student的增删改查。以下是一个简单的示例,演示如何使用JdbcTemplate、Spring和Spring MVC实现学生表的增删改查。
首先,你需要按照上述提到的步骤配置数据库连接和创建`Student`类。
接下来,你可以创建一个`StudentController`类来处理学生表的增删改查请求:
```java
@Controller
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping("/list")
public String getAllStudents(Model model) {
List<Student> students = studentService.getAllStudents();
model.addAttribute("students", students);
return "studentList";
}
@GetMapping("/{id}")
public String getStudentById(@PathVariable int id, Model model) {
Student student = studentService.getStudentById(id);
model.addAttribute("student", student);
return "studentDetail";
}
@GetMapping("/add")
public String showAddForm(Model model) {
Student student = new Student();
model.addAttribute("student", student);
return "addStudentForm";
}
@PostMapping("/add")
public String addStudent(@ModelAttribute("student") Student student) {
studentService.addStudent(student);
return "redirect:/student/list";
}
@GetMapping("/{id}/edit")
public String showEditForm(@PathVariable int id, Model model) {
Student student = studentService.getStudentById(id);
model.addAttribute("student", student);
return "editStudentForm";
}
@PostMapping("/{id}/edit")
public String updateStudent(@PathVariable int id, @ModelAttribute("student") Student student) {
student.setId(id);
studentService.updateStudent(student);
return "redirect:/student/list";
}
@GetMapping("/{id}/delete")
public String deleteStudent(@PathVariable int id) {
studentService.deleteStudent(id);
return "redirect:/student/list";
}
}
```
在上述代码中,`StudentController`类使用`StudentService`来处理学生表的增删改查操作,并通过Spring MVC的注解来定义不同的请求处理方法。
接下来,你可以创建一个`StudentService`类来实现学生表的增删改查逻辑:
```java
@Service
public class StudentService {
@Autowired
private StudentDao studentDao;
public List<Student> getAllStudents() {
return studentDao.getAllStudents();
}
public Student getStudentById(int id) {
return studentDao.getStudentById(id);
}
public void addStudent(Student student) {
studentDao.addStudent(student);
}
public void updateStudent(Student student) {
studentDao.updateStudent(student);
}
public void deleteStudent(int id) {
studentDao.deleteStudent(id);
}
}
```
在上述代码中,`StudentService`类调用`StudentDao`接口中定义的方法来实现学生表的增删改查操作。
最后,你可以使用Spring的配置文件来配置JdbcTemplate和其他相关的组件。假设你使用的是XML配置方式,你可以创建一个名为`applicationContext.xml`的配置文件,并添加以下配置:
```xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 配置数据库连接 -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/db_name" />
<property name="username" value="db_username" />
<property name="password" value="db_password" />
</bean>
<!-- 配置JdbcTemplate -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource" />
</bean>
<!-- 扫描注解 -->
<context:component-scan base-package="com.example.yourpackage" />
</beans>
```
在上述配置中,你需要将`com.example.yourpackage`替换为你实际的包名。
以上是一个简单的示例,你可以根据你的实际需求进行适当的修改和扩展。希望对你有所帮助!
相关推荐









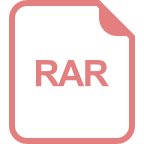
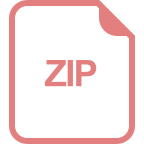