有两个url是文件地址,java如何将其打包成压缩包,然后转成blob流返回前端呢
时间: 2024-09-29 15:11:42 浏览: 22
在Java中,你可以使用Apache Commons IO库来创建一个压缩文件,然后将其转换为Blob对象。以下是步骤:
1. 添加依赖:首先,你需要在项目中添加Apache Commons IO库的依赖。如果你使用Maven,可以在pom.xml文件中添加:
```xml
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.7</version>
</dependency>
```
2. 创建压缩文件:通过`FileUtils`类的`compressDirectoryToZip()`方法,将包含两个文件URL的目录压缩成.zip文件。例如:
```java
import org.apache.commons.io.FileUtils;
import java.io.*;
public byte[] createCompressedArchive(String dirUrl1, String dirUrl2) {
try (InputStream in = new URL(dirUrl1).openStream();
InputStream in2 = new URL(dirUrl2).openStream()) {
File tempDir = File.createTempFile("temp", ".zip");
tempDir.deleteOnExit();
// 获取临时目录路径
String tempPath = tempDir.getAbsolutePath();
// 创建压缩文件
FileUtils.copyURLToFile(in, new File(tempPath, "file1.txt"));
FileUtils.copyURLToFile(in2, new File(tempPath, "file2.txt"));
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(tempPath));
// 将目录内容添加到压缩流
FileSet fileSet = new FileSet(tempDir);
fileSet.setInclude("**/*");
fileSet.setExcludes("META-INF/");
fileSet.copyTo(zos);
zos.close();
byte[] compressedData = Files.readAllBytes(Paths.get(tempPath));
return compressedData;
} catch (IOException e) {
throw new RuntimeException("Failed to compress files", e);
}
}
```
3. 转换为Blob:最后,将压缩后的字节数组转换为Blob对象,可以用于HTTP响应:
```java
import javax.servlet.http.HttpServletResponse;
public void sendCompressedAsBlob(HttpServletResponse response, byte[] data) {
response.setContentType("application/zip");
response.setHeader("Content-Disposition", "attachment; filename=compressed_files.zip");
response.getOutputStream().write(data);
response.flushBuffer();
}
```
相关推荐
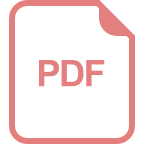
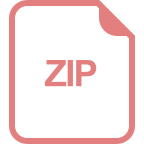
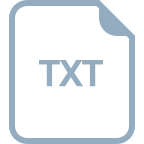
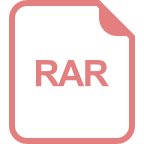
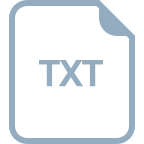
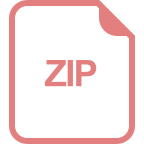
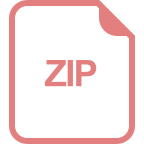
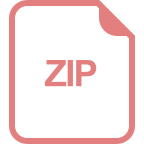
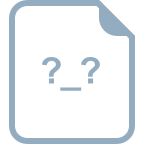
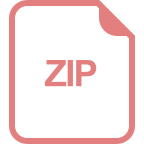
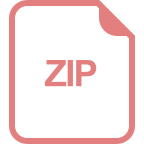
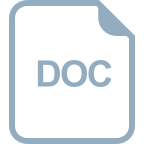
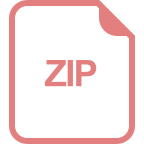