java使用poi删除word表格并在原位置生成表格
时间: 2024-11-23 22:39:38 浏览: 6
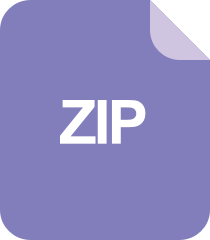
java使用poi操作.doc word模板替换,循环插入表格

在Java中,你可以使用Apache POI库来处理Microsoft Word文档中的表格。POI是一个功能强大的API,可以读取、写入和修改Office文件格式,包括Word (.docx)。如果你想删除Word表格并替换为新的表格,可以按照以下步骤操作:
1. **添加依赖**:
首先,你需要将Apache POI(尤其是`poi-ooxml`和`poi-xwpf.usermodel`包)添加到你的项目中。如果你使用Maven,可以在pom.xml文件中添加相应的依赖。
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>最新版本号</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-xwpf.usermodel</artifactId>
<version>最新版本号</version>
</dependency>
```
2. **创建`XWPFDocument`对象**:
使用`XWPFDocument`打开你的Word文档。
```java
XWPFDocument document = new XWPFDocument(new FileInputStream("your_word_file.docx"));
```
3. **查找和删除表格**:
使用`XWPFTable`类的`getAllTables()`方法获取所有的表格,然后遍历并找到你想要删除的那一个。
```java
for (XWPFTable table : document.getTables()) {
// 根据需要检查表标题或其他识别信息来定位表格
if (table.getTitle().equals("Your Table Title")) {
table.remove();
}
}
```
4. **插入新表格**:
创建一个新的`XWPFTable`实例,并设置其大小和样式。然后将其插入到原来删除表格的位置。
```java
// 创建新表格
XWPFTable newTable = document.createTable(3, 2); // 3行2列
newTable.setTitle("New Table"); // 设置新标题
// 插入新表格到文档的指定位置
int position = ... // 获取之前删除的表格的位置索引
document.insertTable(position, newTable);
```
5. **保存文档**:
最后,将修改后的文档保存回磁盘。
```java
try (OutputStream outputStream = new FileOutputStream("modified_word_file.docx")) {
document.write(outputStream);
} catch (IOException e) {
e.printStackTrace();
}
```
阅读全文
相关推荐
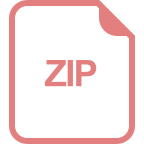
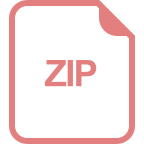


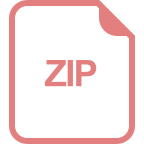
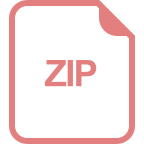
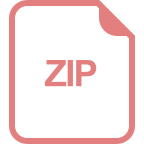
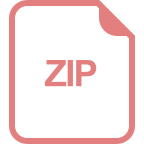
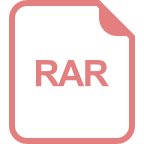
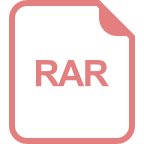
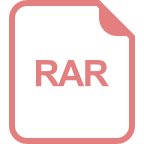
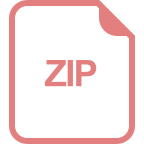



