如何用C++编程实现从用户输入一个字母并转换为其对应的大小写形式?
时间: 2024-12-18 17:18:43 浏览: 9
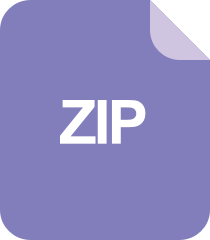
C、C++实现字母/英文句子大小写转换
在C++中,你可以通过标准库中的`<cctype>`头文件提供的函数来实现字符大小写的转换。下面是一个简单的示例:
1. **获取用户输入**:
```cpp
#include <iostream>
#include <cctype> // 包含用于处理字符的头文件
int main() {
char input;
std::cout << "请输入一个字母: ";
std::cin >> input; // 获取用户的输入
}
```
2. **转换大小写**:
```cpp
// 转换为大写
if (islower(input)) { // 判断是否为小写字母
input = toupper(input); // 使用toupper函数转为大写
}
// 转换为小写
else if (isupper(input)) { // 判断是否为大写字母
input = tolower(input); // 使用tolower函数转为小写
}
std::cout << "转换后的字母是: " << input << std::endl;
```
3. **完整代码**:
```cpp
#include <iostream>
#include <cctype>
int main() {
char input;
std::cout << "请输入一个字母: ";
std::cin >> input;
if (islower(input)) {
input = toupper(input);
} else if (isupper(input)) {
input = tolower(input);
}
std::cout << "转换后的字母是: " << input << std::endl;
return 0;
}
```
阅读全文
相关推荐
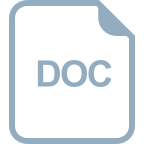
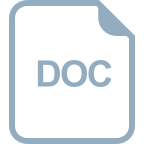
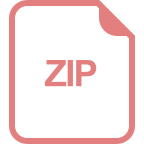
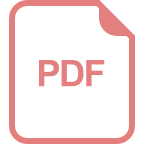
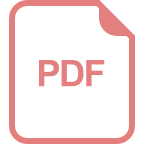
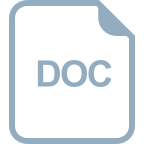

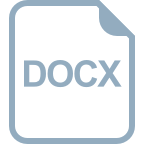
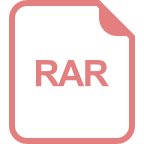
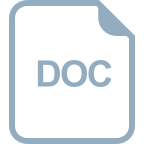
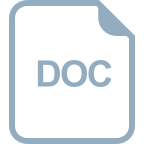
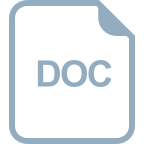
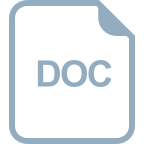




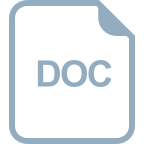