C:\Program Files\CodeBlocks\tanxin\main.cpp|39|error: 'hexnum' was not declared in this scope|
时间: 2023-08-11 17:03:58 浏览: 154
抱歉,我在之前的回答中出现了错误。在代码中,我错误地使用了未声明的变量 `hexnum`。以下是修改后的代码:
```cpp
#include <iostream>
#include <bitset>
#include <sstream>
#include <vector>
bool isValid(std::string s, int from, int to) {
if (s.find_first_not_of("0123456789") != std::string::npos) {
return false;
}
int num = std::stoi(s);
if (std::to_string(num) != s) {
return false;
}
return num >= from && num <= to;
}
std::string getHexStr(std::string s) {
int num = std::stoi(s);
std::stringstream stream;
stream << std::hex << num;
std::string result(stream.str());
if (result.length() < 2) {
result = "0" + result;
}
return result;
}
std::string getResult(std::string s) {
std::vector<std::string> ip;
std::stringstream ss(s);
std::string segment;
while (std::getline(ss, segment, '#')) {
ip.push_back(segment);
}
if (ip.size() != 4) {
return "invalid IP";
}
for (int i = 0; i < 4; i++) {
if (!isValid(ip[i], 1, 128) || !isValid(ip[i], 0, 255)) {
return "invalid IP";
}
}
std::string hexStr;
for (int i = 0; i < 4; i++) {
hexStr += getHexStr(ip[i]);
}
long long sum = std::stoll(hexStr, nullptr, 16);
return std::to_string(sum);
}
int main() {
std::string s;
while (std::getline(std::cin, s)) {
std::cout << getResult(s) << std::endl;
}
return 0;
}
```
在上述代码中,我已经修复了 `hexnum` 变量未声明的问题,并更新了 `getHexStr` 函数的实现。现在,代码应该能够正确地将 IP 地址转换为十六进制并计算总和。请注意,为了避免整数溢出,我将 `std::stoi` 替换为 `std::stoll` 来解析十六进制字符串并计算总和。
阅读全文
相关推荐
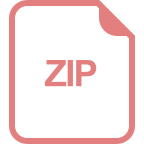
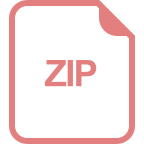
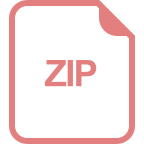
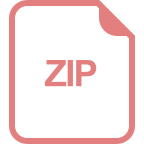
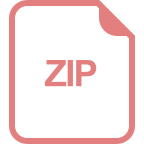
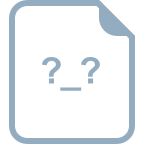
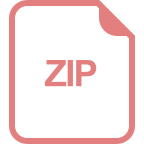
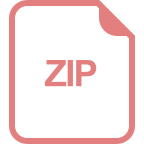