Jupyter Notebook手写数字字母识别完整代码
时间: 2024-07-15 12:00:26 浏览: 181
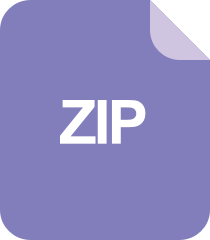
HandWrittenDigitRecognition:Jupyter Notebook识别手写数字
在Jupyter Notebook中实现手写数字和字母识别通常涉及到深度学习和图像处理,特别是使用卷积神经网络(CNN)和库如TensorFlow或PyTorch。以下是一个简化的步骤概述,不包含完整的代码,但你可以根据这个指南在实际环境中编写:
1. **导入必要的库**:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
```
2. **加载MNIST数据集**:
```python
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
```
3. **预处理数据**:
- 将像素值归一化到0-1之间
- reshape为(批量大小, 行, 列, 原始通道数)
```python
train_images = train_images.reshape(-1, 28, 28, 1).astype('float32') / 255.0
test_images = test_images.reshape(-1, 28, 28, 1).astype('float32') / 255.0
```
4. **构建模型**:
```python
model = Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(62, activation='softmax') # 62 for MNIST digits and uppercase letters
])
```
5. **编译和训练模型**:
```python
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=10, validation_data=(test_images, test_labels))
```
6. **评估模型**:
```python
_, test_accuracy = model.evaluate(test_images, test_labels)
print(f"Test accuracy: {test_accuracy}")
```
7. **使用模型进行预测**:
```python
prediction = model.predict(test_images[:1])
predicted_class = np.argmax(prediction)
print(f"Predicted class: {predicted_class}")
```
如果你想要识别字母而不是数字,你可能需要调整最后一层的节点数(从62改为字母的数量),并更新标签。
阅读全文
相关推荐
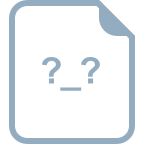
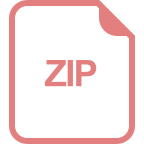


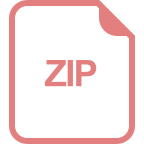
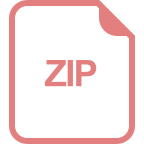
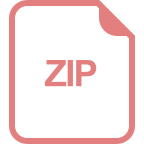
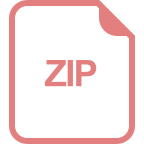
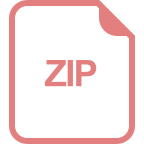
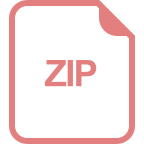
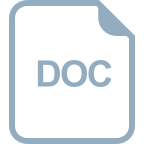
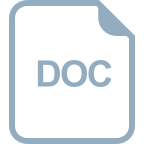
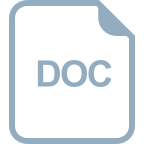
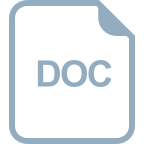
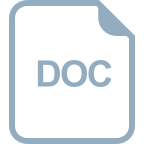
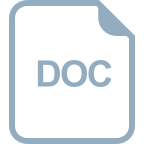
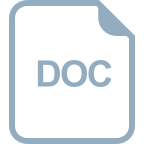
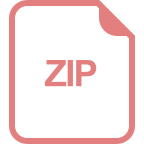
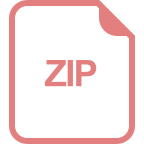