反复读取文件夹中的每张图片的rgb信息,每张图片建立一个对应的txt来保存读取到的rgb信息,保存之前删除对应的txt文本内容。c++实现不使用opencv
时间: 2024-04-14 11:25:53 浏览: 446
要实现这个功能,你可以使用C++的标准库来处理文件和图像。以下是一个基本的实现示例:
```cpp
#include <iostream>
#include <fstream>
#include <filesystem>
namespace fs = std::filesystem;
// 读取图片的RGB信息,并保存到对应的txt文件中
void processImage(const std::string& imagePath) {
// 打开图片文件
std::ifstream imageFile(imagePath, std::ios::binary);
if (!imageFile) {
std::cerr << "Failed to open image file: " << imagePath << std::endl;
return;
}
// 获取图片的大小
imageFile.seekg(0, std::ios::end);
std::streampos imageSize = imageFile.tellg();
imageFile.seekg(0, std::ios::beg);
// 分配内存保存图片数据
char* imageData = new char[imageSize];
imageFile.read(imageData, imageSize);
// 关闭图片文件
imageFile.close();
// 解析图片的RGB信息
std::string rgbInfo;
for (std::streampos i = 0; i < imageSize; i += 3) {
unsigned char r = imageData[i];
unsigned char g = imageData[i + 1];
unsigned char b = imageData[i + 2];
rgbInfo += std::to_string(r) + " " + std::to_string(g) + " " + std::to_string(b) + "\n";
}
// 获取图片文件名(不包含扩展名)
std::string imageFileName = fs::path(imagePath).stem();
// 构建对应的txt文件路径
std::string txtFilePath = imageFileName + ".txt";
// 删除已存在的txt文件
if (fs::exists(txtFilePath)) {
fs::remove(txtFilePath);
}
// 创建并打开txt文件
std::ofstream txtFile(txtFilePath);
if (!txtFile) {
std::cerr << "Failed to create txt file: " << txtFilePath << std::endl;
return;
}
// 写入RGB信息到txt文件
txtFile << rgbInfo;
// 关闭txt文件
txtFile.close();
// 释放内存
delete[] imageData;
}
int main() {
// 图片文件夹路径
std::string folderPath = "path/to/your/folder";
// 遍历文件夹中的图片文件
for (const auto& entry : fs::directory_iterator(folderPath)) {
std::string imagePath = entry.path().string();
// 处理每张图片
processImage(imagePath);
}
return 0;
}
```
请将代码中的 "path/to/your/folder" 替换为要读取图片的文件夹的实际路径。此代码将遍历指定文件夹中的所有图片文件,读取每张图片的RGB信息,并将其保存到对应的txt文件中。如果txt文件已存在,则会先删除旧的txt文件。
阅读全文
相关推荐
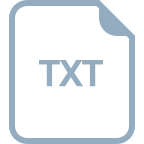
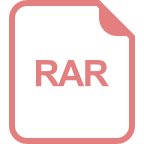
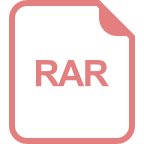
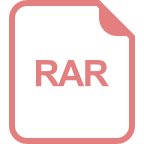
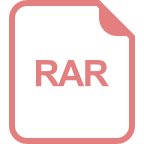
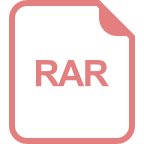
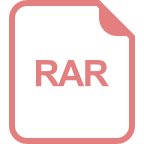
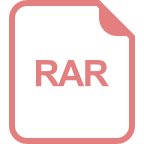
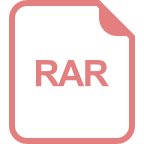
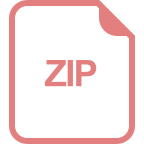
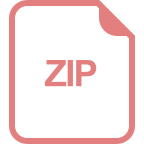
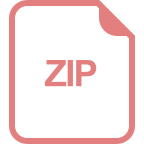