Interop.Illustrator.dll中Illustrator.Application.Visible为只读,如何设置为无界面模式
时间: 2024-12-24 09:41:05 浏览: 6
在Interop.Illustrator.dll中,`Application.Visible`属性确实是只读的,这意味着你无法直接将其设置为`false`来让Illustrator保持无界面模式。不过,你可以通过创建一个新的`Document`对象并设置其`Visible`属性为`false`间接达到类似的效果,因为新文档会启动无界面工作区。
以下是示例代码:
```csharp
using Adobe.Interop.Illustrator;
// 创建一个新的无界面文档
Illustrator.Document invisibleDoc = new Illustrator.Document();
invisibleDoc.Visible = false;
// 然后,你可以像正常一样使用这个无界面文档对象,例如打开AI文件:
invisibleDoc.Open("path_to_your_ai_file.ai");
// ... 进行操作 ...
// 关闭文档后,无界面模式仍然有效
invisibleDoc.Close(SaveOptions.DoNotSave);
// 主程序中,无需显示主应用窗口
Illustrator.Application app = new Illustrator.Application();
app.Visible = false; // 主应用窗口仍为不可见
```
在这种情况下,`app.Visible`依然设为`false`,但实际上所有交互都在`invisibleDoc`上进行,实现了无界面的操作过程。
相关问题
C#中使用Interop.Illustrator.dll保存文件为SVG格式文件代码示例
在C#中,如果你想要通过Interop.Illustator.dll(Illustrator COM Interop)库将Adobe Illustrator文档保存为SVG(Scalable Vector Graphics)格式,你需要首先引用这个库,并确保已经安装了Adobe Illustrator。下面是一个简单的示例代码片段:
```csharp
using Illustrator = Interop.Illustrator;
using System.Runtime.InteropServices;
public void SaveAsSvg(AssociatedGraphicsObject ago)
{
// 创建Illustrator.Application实例
Illustrator.Application app = new Illustrator.Application();
try
{
// 检查是否已打开文档
if (app.ActiveDocument != null)
{
// 获取当前文档的图形对象
Illustrator.Document doc = app.ActiveDocument;
// 设置SVG导出选项
Illustrator.exportOptions svgOptions = new Illustrator.exportOptions();
svgOptions.formatType = Illustrator.exportFormat.aiExportSVG;
svgOptions.embedColorProfiles = true; // 如果需要嵌入颜色配置文件
// 定义保存路径和文件名
string filePath = "path_to_your_svg_file.svg";
// 导出为SVG
doc.Export(filePath, svgOptions);
Console.WriteLine($"SVG文件已成功保存至: {filePath}");
}
else
{
throw new Exception("未打开文档");
}
}
catch (COMException ex)
{
Console.WriteLine($"遇到错误: {ex.Message}");
}
finally
{
// 关闭应用程序
Marshal.ReleaseComObject(app);
}
}
// 调用方法时传入相关的图形对象
AssociatedGraphicsObject myGraphic = ...; // 获取到的图形对象
SaveAsSvg(myGraphic);
```
请注意,这个例子假设你已经有了`AssociatedGraphicsObject`,这是Illustrator API中的一个对象,代表了一个图形实体。实际应用中,你需要处理如何获取这个对象的过程。
Interop.Illustrator.dll获取文件中所有的文本和线段
Interop.Illustrator.dll 是 Adobe Illustrator 的 COM 组件的.NET 版本,它允许开发者通过编程接口(API)从 Illustrator 文件中访问内容,包括文本和路径(线条)。如果你想要获取Illustrator文档中的所有文本和线段,你可以按照以下步骤操作:
1. 引入命名空间:首先,你需要添加 `System.Runtime.InteropServices` 和 `Adobe.Illustrator.Interop` 命名空间。
```csharp
using System.Runtime.InteropServices;
using Adobe.Illustrator.Interop;
```
2. 创建 Illustrator 对象:通过 `Illustrator.ApplicationClass` 初始化 Illustrator 应用程序实例。
```csharp
var app = new Illustrator.Application();
```
3. 打开文件:使用 `app.Documents.Open()` 方法打开需要处理的文件。
4. 遍历文档中的所有图形:遍历每个页面(`Document.Pages`),然后检查每个图形(`Item`)是否是文本或路径。如果是,添加到相应的列表中。
```csharp
List<GraphicsItem> texts = new List<GraphicsItem>();
List<GraphicsItem> lines = new List<GraphicsItem>();
foreach (var page in app.ActiveDocument.Pages)
{
foreach (var item in page.Items)
{
if (item is Illustrator.TextItem textItem)
texts.Add(textItem);
else if (item is Illustrator.PolygonPathItem lineItem)
lines.Add(lineItem);
}
}
```
5. 关闭应用程序:处理完文件后别忘了关闭它。
```csharp
app.Quit();
```
阅读全文
相关推荐
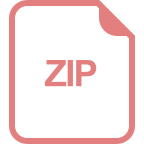
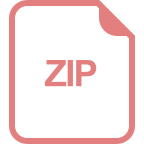
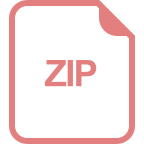













