jupyter notebook画多因子数据图
时间: 2023-10-09 22:14:17 浏览: 155
要在Jupyter Notebook中绘制多因子数据图,你可以使用Python的matplotlib库。下面是一个简单的示例代码,展示如何使用matplotlib来绘制多因子数据图:
```python
import matplotlib.pyplot as plt
# 假设你有两个因子数据,分别是factor1和factor2
factor1 = [1, 2, 3, 4, 5]
factor2 = [5, 4, 3, 2, 1]
# 绘制因子数据图
plt.plot(factor1, label='Factor 1')
plt.plot(factor2, label='Factor 2')
# 添加图例
plt.legend()
# 添加标题和坐标轴标签
plt.title('Multiple Factor Data')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# 显示图像
plt.show()
```
这段代码首先导入了matplotlib库,并创建了两个因子数据列表factor1和factor2。然后使用`plt.plot()`函数绘制因子数据图,并使用`label`参数为每个因子添加标签。接下来使用`plt.legend()`函数添加图例。然后使用`plt.title()`函数添加图表标题,`plt.xlabel()`和`plt.ylabel()`函数分别添加X轴和Y轴的标签。最后使用`plt.show()`函数显示图像。
希望这个例子能帮助你绘制多因子数据图。如果你有任何其他问题,请随时提问。
相关问题
数据包含以下因子:国家/宗教,出生年份,年龄,性别。根据这些信息,如何使用Jupyter Notebook绘制一张人口金字塔图
要绘制人口金字塔图,可以按照以下步骤操作:
1. 在Jupyter Notebook中打开一个新的Notebook文件,在第一行输入以下代码以导入必要的库:
```python
import pandas as pd
import matplotlib.pyplot as plt
```
2. 接下来,创建一个包含人口数据的Pandas DataFrame,其中包含以下列:国家/宗教,出生年份,年龄和性别。例如:
```python
data = {'Country/Religion': ['USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA'],
'Year of Birth': [2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005],
'Age': [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22],
'Gender': ['M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M']}
df = pd.DataFrame(data)
```
3. 使用Pandas的groupby()函数将数据按性别分组,并计算每个年龄组的人口数量。
```python
male_data = df[df['Gender']=='M'].groupby('Age').size()
female_data = df[df['Gender']=='F'].groupby('Age').size()
```
4. 使用Matplotlib绘制人口金字塔图。首先,创建一个新的Figure对象,并添加两个子图,一个用于男性数据,一个用于女性数据。接下来,使用barh()函数绘制水平条形图。
```python
fig, (ax1, ax2) = plt.subplots(ncols=2, sharey=True, figsize=(8, 5))
ax1.barh(male_data.index, male_data.values, align='center', color='blue')
ax2.barh(female_data.index, -female_data.values, align='center', color='red')
```
5. 最后,添加标签和标题,并显示图表。
```python
ax1.set(title='Male', xlabel='Number of people')
ax2.set(title='Female', xlabel='Number of people', xlim=[-max(female_data.values)-100, max(male_data.values)+100])
ax1.set_ylabel('Age')
plt.show()
```
完整的代码如下:
```python
import pandas as pd
import matplotlib.pyplot as plt
data = {'Country/Religion': ['USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA', 'USA'],
'Year of Birth': [2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005, 2005],
'Age': [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22],
'Gender': ['M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M', 'M']}
df = pd.DataFrame(data)
male_data = df[df['Gender']=='M'].groupby('Age').size()
female_data = df[df['Gender']=='F'].groupby('Age').size()
fig, (ax1, ax2) = plt.subplots(ncols=2, sharey=True, figsize=(8, 5))
ax1.barh(male_data.index, male_data.values, align='center', color='blue')
ax2.barh(female_data.index, -female_data.values, align='center', color='red')
ax1.set(title='Male', xlabel='Number of people')
ax2.set(title='Female', xlabel='Number of people', xlim=[-max(female_data.values)-100, max(male_data.values)+100])
ax1.set_ylabel('Age')
plt.show()
```
这样就可以绘制一个简单的人口金字塔图了,具体效果如下图所示:

现有一个人口统计数据文件,其包含以下因子:国家/宗教,出生年份,年龄,性别。根据这些信息,如何使用Jupyter Notebook绘制一张人口金字塔图
要绘制人口金字塔图,可以按照以下步骤操作:
1. 在Jupyter Notebook中打开一个新的Notebook文件,在第一行输入以下代码以导入必要的库:
```python
import pandas as pd
import matplotlib.pyplot as plt
```
2. 从数据文件中读取数据,并创建一个包含人口数据的Pandas DataFrame,其中包含以下列:国家/宗教,出生年份,年龄和性别。例如:
```python
df = pd.read_csv('population.csv')
```
3. 使用Pandas的groupby()函数将数据按性别分组,并计算每个年龄组的人口数量。
```python
male_data = df[df['Gender']=='M'].groupby('Age').size()
female_data = df[df['Gender']=='F'].groupby('Age').size()
```
4. 使用Matplotlib绘制人口金字塔图。首先,创建一个新的Figure对象,并添加两个子图,一个用于男性数据,一个用于女性数据。接下来,使用barh()函数绘制水平条形图。
```python
fig, (ax1, ax2) = plt.subplots(ncols=2, sharey=True, figsize=(8, 5))
ax1.barh(male_data.index, male_data.values, align='center', color='blue')
ax2.barh(female_data.index, -female_data.values, align='center', color='red')
```
5. 最后,添加标签和标题,并显示图表。
```python
ax1.set(title='Male', xlabel='Number of people')
ax2.set(title='Female', xlabel='Number of people', xlim=[-max(female_data.values)-100, max(male_data.values)+100])
ax1.set_ylabel('Age')
plt.show()
```
完整的代码如下:
```python
import pandas as pd
import matplotlib.pyplot as plt
df = pd.read_csv('population.csv')
male_data = df[df['Gender']=='M'].groupby('Age').size()
female_data = df[df['Gender']=='F'].groupby('Age').size()
fig, (ax1, ax2) = plt.subplots(ncols=2, sharey=True, figsize=(8, 5))
ax1.barh(male_data.index, male_data.values, align='center', color='blue')
ax2.barh(female_data.index, -female_data.values, align='center', color='red')
ax1.set(title='Male', xlabel='Number of people')
ax2.set(title='Female', xlabel='Number of people', xlim=[-max(female_data.values)-100, max(male_data.values)+100])
ax1.set_ylabel('Age')
plt.show()
```
这样就可以绘制一个简单的人口金字塔图了,具体效果如下图所示:

阅读全文
相关推荐
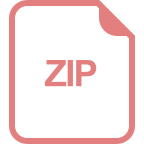
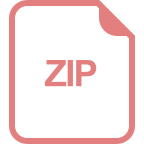
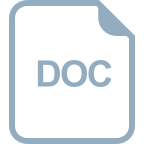
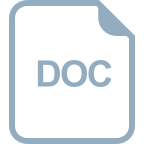
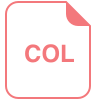

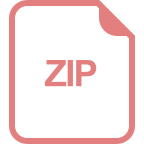
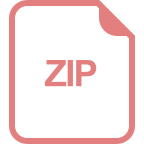
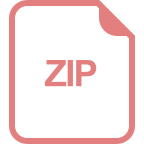
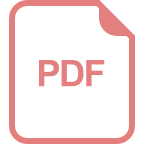
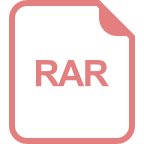
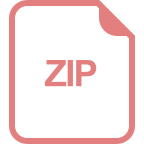
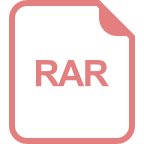
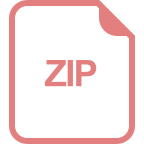
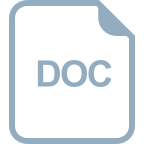
