java用二维数组打印星号正三角形
时间: 2024-10-08 08:09:35 浏览: 26
在Java中,我们可以使用二维数组来打印出星号构成的正三角形。以下是一个简单的例子:
```java
public class Main {
public static void main(String[] args) {
int rows = 5; // 正三角形的行数
printTriangle(rows);
}
public static void printTriangle(int size) {
// 创建一个大小为rows * (rows + 1) / 2 的二维数组
char[][] triangle = new char[size][size*(size+1)/2];
// 使用嵌套循环填充星号
for (int i = 0; i < size; i++) {
for (int j = 0; j <= i; j++) {
triangle[i][j] = '*';
}
System.out.println(triangle[i]);
}
}
}
```
这个程序会打印出如下的5行星号正三角形:
```
*
**
***
****
*****
```
相关问题
用JAVA数组打印等腰三角形
在Java中,你可以使用二维数组或一维数组结合循环来打印等腰三角形。这里是一个简单的例子,使用一维数组来演示如何打印一个基础的等腰三角形:
```java
public class Main {
public static void main(String[] args) {
int n = 5; // 三角形的行数
printTriangle(n);
}
public static void printTriangle(int rows) {
for (int i = 1; i <= rows; i++) {
// 打印空格
for (int j = rows - i; j > 0; j--) {
System.out.print(" ");
}
// 打印星号
for (int k = 1; k <= 2 * i - 1; k++) {
System.out.print("*");
}
// 换行
System.out.println();
}
// 为了美观,可以打印最后一行多余的空格
for (int l = rows - 1; l >= 0; l--) {
System.out.print(" ");
}
}
}
```
在这个例子中,`printTriangle`函数接受行数作为参数,外层循环控制每一行的开始,内层循环分别用于打印左侧的空格和右侧的星号。每增加一行,星号的数量就会相应增加。
Java中除了星号还能用什么符号打印图形?
在Java中,除了星号(*)之外,还可以使用其他字符如逗号`,`、竖线`|`、感叹号`!`、下划线 `_` 等来打印图形。比如,你可以使用ASCII艺术或者自定义字符数组来构建复杂的二维图形。每个字符的位置和组合都可以用来表示特定的形状或图案。
例如,绘制简单的水平线或垂直线就可以用到竖线`|`,而使用连字符`-`可以创建等宽的线条。对于更复杂的设计,可能会涉及到字符串连接或者矩阵操作。
下面是一个使用竖线和空格创建简单直角三角形的例子:
```java
public class Main {
public static void main(String[] args) {
int height = 5;
for (int i = 0; i < height; i++) {
for (int j = 0; j <= i; j++) {
System.out.print(j == 0 || j == i ? "|" : " ");
}
System.out.println();
}
}
}
```
这里使用了条件判断来选择是否打印竖线(`|`) 或者空格。
阅读全文
相关推荐
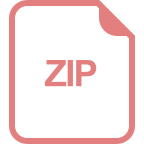
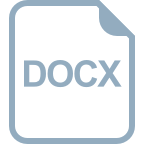
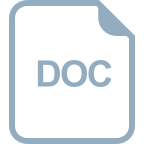
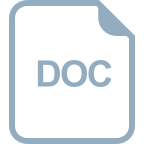
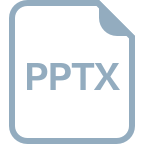
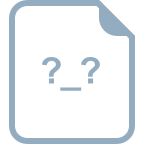
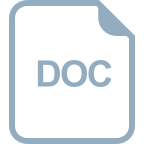
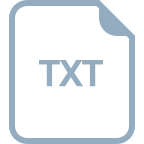
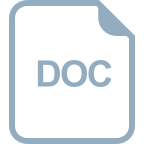
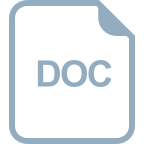
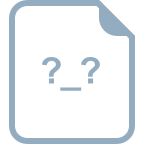
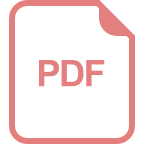
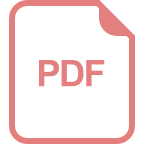
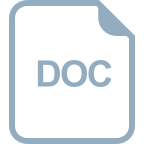
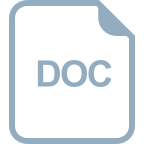
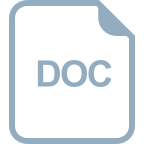
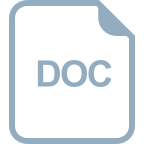