用C#写ftp文件传输程序
时间: 2024-10-12 22:17:06 浏览: 37
在C#中编写FTP文件传输程序通常涉及到使用第三方库,如FtpClient或FluentFTP,因为标准库并不直接提供FTP功能。以下是一个基本的使用FluentFTP库的例子:
首先,你需要安装FluentFTP库,你可以通过NuGet包管理器添加:
```csharp
Install-Package FluentFTP;
```
然后,可以编写一个简单的上传文件的函数:
```csharp
using FluentFTP;
public async Task UploadFileToFtp(string localFilePath, string remoteDirectory, string ftpServerAddress, string username, string password)
{
using (var client = new FtpClient(ftpServerAddress))
{
try
{
await client.ConnectAsync(username, password); // 连接到FTP服务器
await client.AuthenticateAsync(FtpAuthenticationType.BASIC); // 认证
await client.ChangeWorkingDirectoryAsync(remoteDirectory); // 切换到目标目录
await client.UploadAsync(localFilePath, remoteFilePath); // 上传文件
await client.DisconnectAsync(); // 断开连接
}
catch (FtpException ex)
{
Console.WriteLine($"Error uploading file: {ex.Message}");
}
}
}
```
使用此函数,只需传递本地文件路径、远程目录地址、FTP服务器地址以及用户名和密码即可:
```csharp
await UploadFileToFtp(@"C:\path\to\local\file.txt", "/remote/directory/", "username", "password");
```
阅读全文
相关推荐
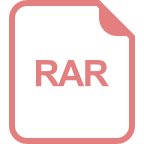
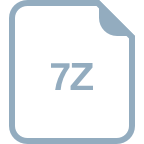
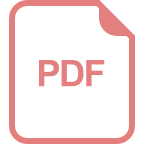

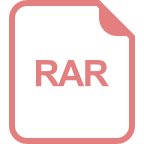
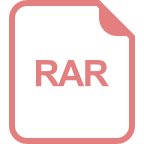
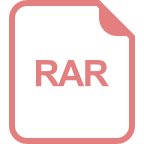
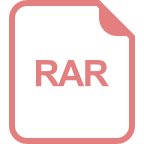
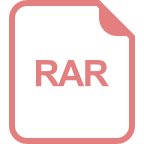
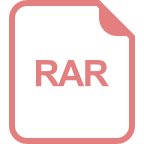
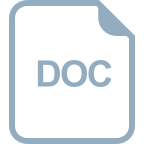
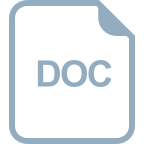
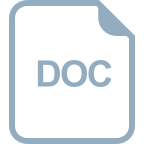
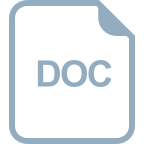
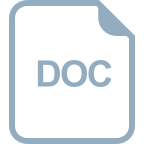
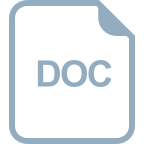
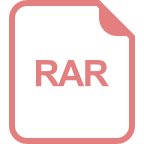
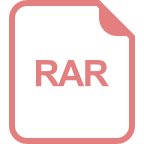
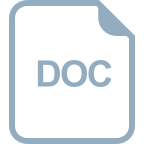