如何编写一个程序来计算两点间的长度?
时间: 2024-11-13 08:42:21 浏览: 13
要编写一个程序来计算两点之间的距离,首先我们需要知道两点的坐标通常是以笛卡尔坐标的形式表示的,即(x1, y1) 和 (x2, y2),其中 x 是水平轴上的值,y 是垂直轴上的值。两点间距离的计算公式是欧几里得距离公式:
\[
d = \sqrt{(x2 - x1)^2 + (y2 - y1)^2}
\]
在 C++ 中,你可以这样做:
```cpp
#include <iostream>
#include <cmath> // 引入数学库,用于 sqrt 函数
class Point {
public:
double x;
double y;
// 构造函数
Point(double x = 0, double y = 0) : x(x), y(y) {}
// 计算两点间的距离
double distance(const Point& other) const {
return std::sqrt(std::pow(other.x - this->x, 2) + std::pow(other.y - this->y, 2));
}
};
int main() {
Point pointA(3, 4); // 假设这是第一个点 (3, 4)
Point pointB(6, 8); // 假设这是第二个点 (6, 8)
double distanceAB = pointA.distance(pointB);
std::cout << "The distance between points A and B is: " << distanceAB << std::endl;
return 0;
}
```
在这个例子中,我们定义了一个名为 `Point` 的类,包含两个私有变量 `x` 和 `y` 代表点的坐标。然后我们提供了一个 `distance` 成员函数,接受另一个 `Point` 类型的参数并返回两点之间的距离。
在 `main` 函数中,我们创建了两个 `Point` 对象并调用它们的距离方法来计算距离。
阅读全文
相关推荐
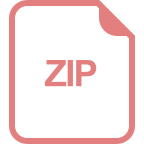
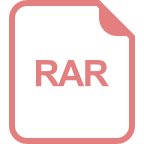
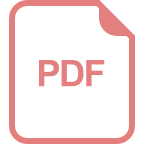
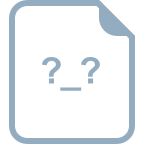
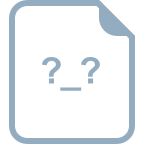
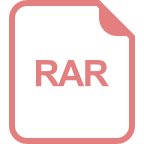
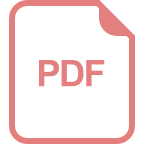
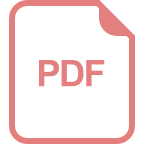
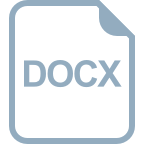
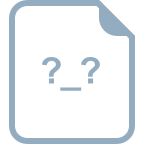
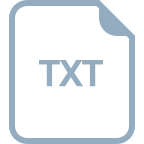
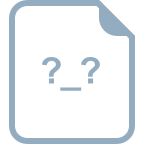
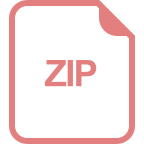
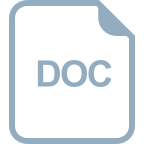
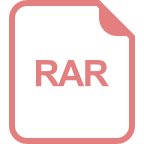
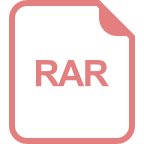
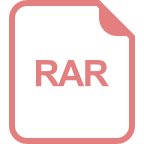
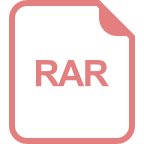
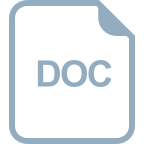