给我出几道c++ do while的练习题
时间: 2024-09-29 08:04:48 浏览: 40
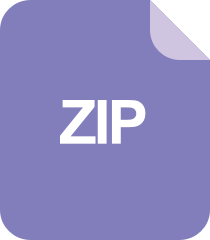
蓝桥杯c++-蓝桥杯竞赛练习之基础练习题数列特征.zip
当然可以。`do-while`循环在C++中通常用于至少执行一次循环体,然后根据条件判断是否继续。这里有一些简单的`do-while`循环练习题供你参考:
1. **无限计数器**:
编写一个程序,让用户输入数字,直到用户输入'0'为止。每次循环都要打印当前计数,并询问用户是否还要继续。
```cpp
#include <iostream>
int main() {
int count = 0;
do {
std::cout << "当前计数: " << count << std::endl;
std::cin >> count;
} while (count != 0);
return 0;
}
```
2. **猜数字游戏**:
写一个程序,生成一个随机数,让玩家猜测。如果猜错了,会告诉他们是猜大了还是猜小了,直到猜对为止。`do-while`在这种情况下可以保证至少询问一次。
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(time(0)); // 初始化随机数种子
int secret = rand() % 100 + 1; // 随机数范围从1到100
int guess;
do {
std::cout << "请输入你猜的数字(1-100):";
std::cin >> guess;
} while (guess != secret);
std::cout << "恭喜你,猜对了!" << std::endl;
return 0;
}
```
3. **字符检测**:
创建一个程序,读取用户输入直到遇到换行符,然后显示用户输入了多少次回车和空格。
```cpp
#include <iostream>
#include <string>
std::string input;
int enter_count = 0, space_count = 0;
void processInput() {
if (input.back() == '\n') {
// 如果是换行符,则计算统计值并清空字符串
enter_count++;
input.pop_back();
}
else if (input.back() == ' ') {
space_count++;
input.pop_back();
}
}
int main() {
do {
std::getline(std::cin, input);
} while (!input.empty());
std::cout << "换行次数: " << enter_count << ", 空格次数: " << space_count << std::endl;
return 0;
}
```
阅读全文
相关推荐
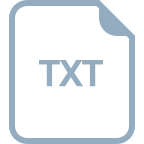
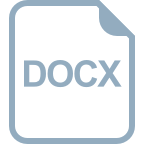

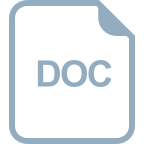
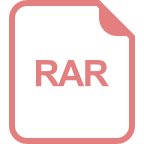
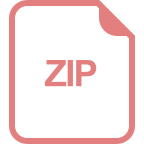
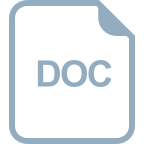
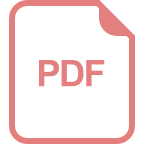
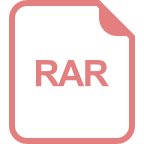
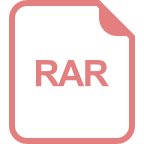
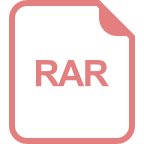
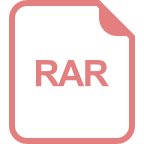
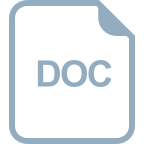
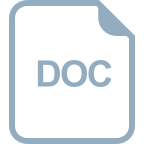


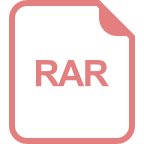