编写两个进程,一个负责输入,一个输出对应的回文数,要用重定向到第三个文件,linux下用c怎么写,命名管道
时间: 2024-10-20 12:04:12 浏览: 22
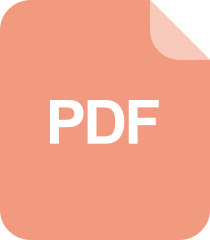
PHP输出两个数字中间有多少个回文数的方法
在Linux下,你可以使用命名管道(也称为FIFO,即First In First Out)来实现两个进程之间的通信,其中一个进程用于输入,另一个负责处理并输出回文数。这里是一个简单的示例,如何使用C语言编写:
首先,创建一个FIFO:
```bash
mkfifo input_pipe
```
然后,创建两个C程序,一个名为`input_reader.c`,用于读取标准输入并写入FIFO:
```c
#include <stdio.h>
#include <unistd.h>
void write_to_fifo(int fd) {
char buffer[100]; // 这里假设输入长度不超过100字符
while (fgets(buffer, sizeof(buffer), stdin)) {
if (write(fd, buffer, strlen(buffer)) == -1) {
perror("Error writing to FIFO");
break;
}
}
close(fd);
}
int main() {
int pipe_fd = open("input_pipe", O_WRONLY); // 输出模式打开FIFO
if (pipe_fd < 0) {
perror("Failed to open FIFO");
return 1;
}
write_to_fifo(pipe_fd);
printf("Input reader finished.\n");
return 0;
}
```
接下来,另一个进程`output_writer.c`从FIFO读取内容并检查是否为回文:
```c
#include <stdio.h>
#include <string.h>
int is_palindrome(const char* str) {
int len = strlen(str);
for (int i = 0; i < len / 2; ++i) {
if (str[i] != str[len - 1 - i]) {
return 0;
}
}
return 1;
}
void read_from_fifo(int fd) {
char buffer[100];
while (read(fd, buffer, sizeof(buffer)) > 0) {
if (is_palindrome(buffer)) {
printf("%s is a palindrome.\n", buffer);
} else {
printf("%s is not a palindrome.\n", buffer);
}
}
close(fd);
}
int main() {
int pipe_fd = open("input_pipe", O_RDONLY); // 输入模式打开FIFO
if (pipe_fd < 0) {
perror("Failed to open FIFO");
return 1;
}
read_from_fifo(pipe_fd);
printf("Output writer finished.\n");
return 0;
}
```
最后,在终端运行这两个程序:
```bash
./input_reader &
./output_writer
```
阅读全文
相关推荐
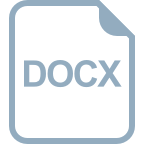
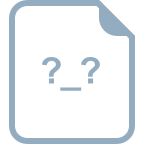
















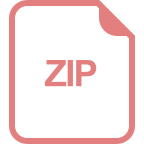