main.py调用detect.py
时间: 2024-09-18 07:06:22 浏览: 42
在Python中,如果你有一个名为`main.py`的主程序文件想要调用另一个名为`detect.py`的脚本,通常的做法是在`main.py`中导入`detect.py`模块,然后按照需要调用其内部的功能。假设`detect.py`包含了一个函数`run_detection()`,你可以这样做:
**main.py:**
```python
import detect # 导入detect.py模块
# 初始化检测模块
detector = detect.DetectClass() # 如果detect.py中有相应类
# 调用detect.py中的run_detection()函数
result = detector.run_detection(some_input参数)
# 执行完检测后,可以对结果进行处理或输出
print("Detection result:", result)
# 更复杂的情况可能涉及到循环调用或者异步操作
while True:
new_input = get_user_input() # 获取用户新输入
result = detector.run_detection(new_input)
process_result(result)
```
在这个例子中,`some_input参数`应替换为你实际需要传递给`run_detection()`函数的数据。如果`detect.py`中的函数需要在主程序外部初始化,记得在`main.py`中进行适当的实例化。
相关问题
main.py调用没有相同类的detect.py
`main.py` 调用 `detect.py` 中的函数或类时,如果两者不在同一个类或者模块里,并且它们之间没有任何形式的导入关联,通常需要通过相对路径或者绝对路径来指定 `detect.py` 的位置。如果你想要从 `main.py` 文件直接访问 `detect.py` 内部的某个功能,例如:
```python
# 假设 detect.py 中有一个公开的函数
def detect_function():
# ... 实现 ...
# 在 main.py 中这样调用
import sys
sys.path.append('/path/to/detect') # 设置正确的目录以便找到 detect.py
from detect import detect_function
detect_function()
```
这里我们首先将 `detect.py` 所在路径添加到 Python 的搜索路径 (`sys.path`) 中,然后通过模块名 `detect` 导入并调用 `detect_function()`。
如果没有相同的类,那么 `detect.py` 可能提供的是函数、类的实例方法或其他公共接口。确保 `detect.py` 提供了可以外部访问的部分,并且 `main.py` 能够正确地引用它。
如何在Yolov8的detect.py文件中添加中心点坐标显示和打印功能,通过找到并修改`box_label`函数和plotting.py中的相关代码实现心点坐标
要在YOLOv8的`detect.py`文件中添加中心点坐标显示和打印功能,你需要对`box_label`函数进行扩展,并在`plotting.py`中的`draw_boxes`函数中加入相应的处理。这里是一个简化的步骤说明:
1. **修改`box_label`函数**:
找到`box_label`函数,通常在`yolov8/detect.py`中的`_get_label_colors`下面。你需要在这个函数返回的字典`labels`中添加一个新的键值对,比如"center_x" 和 "center_y",用于存储每个检测框的中心坐标。
```python
def box_label(boxes, labels, img_size, class_names):
boxes, labels = np.array(boxes), np.array(labels)
labels = [class_names[int(label)] for label in labels]
# 新增中心点坐标
centers = (boxes[:, :2] + boxes[:, 2:]) / 2
return boxes, labels, centers
```
2. **更新`plotting.py`中的`draw_boxes`函数**:
在`draw_boxes`函数中,你可以使用matplotlib或其他绘图库来绘制中心点。例如,可以添加一个新的循环来同时画出边界框和中心点:
```python
def draw_boxes(img, boxes, labels, scores, colors=None, center=True):
if not isinstance(center, bool):
raise ValueError("center should be a boolean indicating whether to display center points")
... # 其他原有代码
if center:
for i in range(len(boxes)):
x, y, w, h = boxes[i]
cx, cy = x + w / 2, y + h / 2
cv2.circle(img, (int(cx), int(cy)), 4, colors[i], -1) # 绘制圆点表示中心点
... # 继续绘制边界框
```
3. **打印中心点**:
如果你想在控制台上打印中心点信息,可以在`main()`函数或`run_nms()`函数中遍历检测结果,获取并打印每一帧的中心点:
```python
for frame_id, result in enumerate(results):
boxes, labels, centers = box_label(result['boxes'], result['labels'], ...)
for i, center in enumerate(centers):
print(f'Frame {frame_id}: Center point ({center[0]:.2f}, {center[1]:.2f}) belongs to class "{labels[i]}"')
... # 继续绘制图像和保存
```
记得在`main()`函数或适当的地方调用`draw_boxes`函数,并确保导入所需的库,如`cv2`和`numpy`。
阅读全文
相关推荐
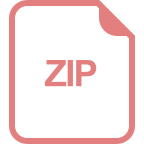
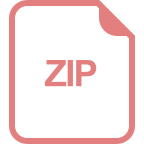
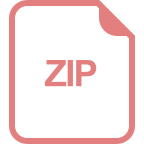








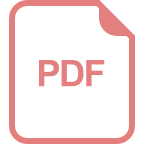
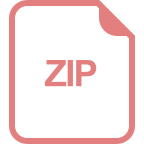
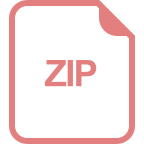
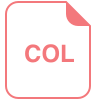
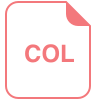
