c++ stack STL
时间: 2024-03-05 19:42:20 浏览: 96
C++中的stack是一种容器适配器,它是使用deque、list或vector实现的。stack提供了后进先出(LIFO)的数据结构,只能在栈顶进行插入和删除操作。它具有以下几个主要的成员函数:
- push(): 在栈顶插入一个元素。
- pop(): 删除栈顶的元素。
- top(): 返回栈顶的元素。
- empty(): 检查栈是否为空。
- size(): 返回栈中元素的个数。
使用stack的时候需要包含<stack>头文件,并且可以通过stack<数据类型>来定义一个特定类型的栈。
相关问题
c++ STL stack
c STL Stack是一个使用List和Vector实现的栈。可以通过包含"心希盼 Stack.doc"来详细了解它的实现方式。
栈的用途之一是进行括号匹配的检验。在使用前可以使用成员函数size()或empty()来检查栈是否为空。例如,可以使用以下代码来检验栈是否为空:
```cpp
#include <iostream>
#include <stack>
using namespace std;
int main(){
stack<int> st;
// 其他操作
if (st.empty()) {
// 栈为空
} else {
// 栈不为空
}
return 0;
}
```
栈的另一个应用是进制转换。例如,可以使用以下代码将一个十进制数转换为指定进制数:
```cpp
#include <iostream>
#include <stack>
using namespace std;
int main(){
int n, x;
stack<int> s;
cin >> n >> x;
while(n){
s.push(n%x);
n = n/x;
}
while(!s.empty()){
int e = s.top();
s.pop();
cout << e;
}
return 0;
}
```
c++stl stack
C++ STL(标准模板库)中的 stack 是一种容器适配器,它提供了一种 LIFO(Last In First Out)数据结构。 stack 可以使用不同的底层容器实现,例如 vector、deque 和 list 等。默认情况下,stack 使用 deque 作为其底层容器。
以下是一些 stack 常用的操作:
1. push():在 stack 的顶部插入一个元素。
2. pop():从 stack 的顶部弹出元素。
3. top():返回 stack 的顶部元素。
4. empty():检查 stack 是否为空。
5. size():返回 stack 中元素的数量。
以下是一个示例代码,展示如何使用 stack:
```
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> s;
s.push(1);
s.push(2);
s.push(3);
while (!s.empty()) {
cout << s.top() << " ";
s.pop();
}
return 0;
}
```
输出结果为:3 2 1。
阅读全文
相关推荐


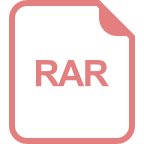
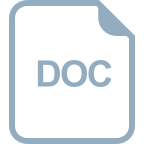
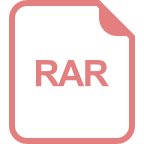




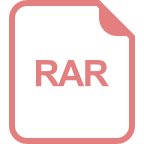


