typescript String去重
时间: 2024-02-22 20:54:09 浏览: 199
在 TypeScript 中,可以使用 Set 数据结构来实现字符串去重。Set 是一种集合数据结构,它只会存储唯一的值,不会重复。以下是一种实现字符串去重的方法:
```typescript
function deduplicateStrings(strings: string[]): string[] {
const uniqueStrings = new Set(strings);
return Array.from(uniqueStrings);
}
const strings = ["apple", "banana", "apple", "orange", "banana"];
const deduplicatedStrings = deduplicateStrings(strings);
console.log(deduplicatedStrings); // Output: ["apple", "banana", "orange"]
```
在上面的代码中,我们定义了一个 `deduplicateStrings` 函数,它接受一个字符串数组作为参数。我们使用 `Set` 数据结构来创建一个存储唯一字符串的集合 `uniqueStrings`。然后,我们将 `uniqueStrings` 转换为数组并返回。
这样,重复的字符串就会被去除,只保留唯一的字符串。
相关问题
帮我用typescript写一个 数组对象根据一个key值去重, key是参数
好的,以下是用 TypeScript 实现的函数:
```typescript
interface Obj {
[key: string]: any;
}
function uniqueByKey<T extends Obj>(arr: T[], key: keyof T): T[] {
const map = new Map<T[keyof T], T>();
arr.forEach((item) => map.set(item[key], item));
return Array.from(map.values());
}
```
这个函数接收两个参数,第一个参数 `arr` 是一个数组,里面的每个元素都是一个对象。第二个参数 `key` 是一个字符串类型的键,表示根据哪个键去重。
函数首先创建了一个 `Map` 对象 `map`,用来存放去重后的结果。然后遍历数组 `arr` 的每个元素,根据 `key` 的值将元素存入 `map` 中。
最后,将 `map` 中所有的值转为数组返回即可。
使用示例:
```typescript
interface Person {
name: string;
age: number;
}
const arr: Person[] = [
{ name: 'Alice', age: 18 },
{ name: 'Bob', age: 20 },
{ name: 'Alice', age: 22 },
{ name: 'Charlie', age: 18 },
];
const result = uniqueByKey(arr, 'name');
console.log(result);
/*
[
{ name: 'Alice', age: 18 },
{ name: 'Bob', age: 20 },
{ name: 'Charlie', age: 18 },
]
*/
```
ts对象数组去重
可以使用`Set`数据结构来进行数组去重。具体操作如下:
```typescript
interface Person {
name: string;
age: number;
}
const persons: Person[] = [
{ name: "Alice", age: 20 },
{ name: "Bob", age: 22 },
{ name: "Alice", age: 20 },
{ name: "Charlie", age: 25 },
];
const uniquePersons = Array.from(new Set(persons.map((p) => JSON.stringify(p)))).map(
(p) => JSON.parse(p)
);
console.log(uniquePersons);
// Output: [{ name: "Alice", age: 20 }, { name: "Bob", age: 22 }, { name: "Charlie", age: 25 }]
```
上述代码中,首先使用`map`将对象数组转化为字符串数组,再使用`Set`去重,最后再将字符串转回对象。注意,这里对对象进行了序列化和反序列化,因此对于对象的属性顺序敏感。如果需要忽略属性顺序,则需要使用其他方式进行去重。
阅读全文
相关推荐
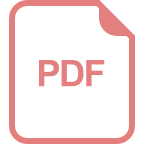
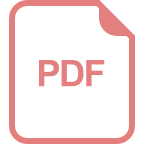
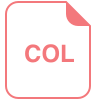

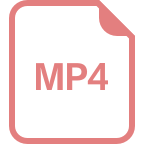
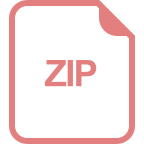
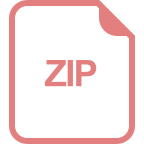
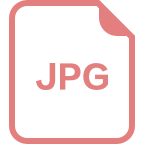