python消消乐小游戏
时间: 2023-11-20 09:59:11 浏览: 47
以下是一个使用Python和pygame库编写的经典小球消消乐游戏的示例:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 480
screen_height = 800
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("消消乐小游戏")
# 加载游戏背景图片
background = pygame.image.load("background.png").convert()
# 加载小球图片
ball_images = []
for i in range(1, 6):
ball_images.append(pygame.image.load("ball" + str(i) + ".png").convert_alpha())
# 设置小球的大小
ball_width = ball_images[0].get_width()
ball_height = ball_images[0].get_height()
# 设置游戏面板大小
board_width = 300
board_height = 50
# 加载游戏面板图片
board_image = pygame.image.load("board.png").convert_alpha()
# 设置游戏面板的位置
board_x = (screen_width - board_width) // 2
board_y = screen_height - board_height - 10
# 设置小球的初始位置
ball_x = random.randint(0, screen_width - ball_width)
ball_y = random.randint(0, screen_height // 2)
# 设置小球的移动速度
ball_speed_x = 5
ball_speed_y = 5
# 设置游戏时钟
clock = pygame.time.Clock()
# 设置游戏分数
score = 0
# 设置游戏结束标志
game_over = False
# 游戏循环
while not game_over:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# 移动小球
ball_x += ball_speed_x
ball_y += ball_speed_y
# 检测小球是否碰到了屏幕边缘
if ball_x < 0 or ball_x > screen_width - ball_width:
ball_speed_x = -ball_speed_x
if ball_y < 0:
ball_speed_y = -ball_speed_y
if ball_y > screen_height - ball_height:
game_over = True
# 绘制游戏背景
screen.blit(background, (0, 0))
# 绘制游戏面板
screen.blit(board_image, (board_x, board_y))
# 绘制小球
screen.blit(ball_images[0], (ball_x, ball_y))
# 更新游戏分数
score_text = "Score: " + str(score)
font = pygame.font.Font(None, 36)
score_image = font.render(score_text, True, (255, 255, 255))
screen.blit(score_image, (10, 10))
# 更新游戏屏幕
pygame.display.update()
# 控制游戏帧率
clock.tick(60)
# 退出游戏
pygame.quit()
```
相关推荐
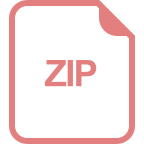
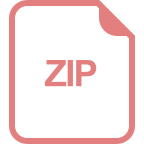














